istream::getline return type
Solution 1
It returns a stream so that we can chain the operation.
But when you use an object in a boolean context the compiler looks for an conversion operator that can convert it into a type that can be used in the boolean context.
C++11
In this case stream has explicit operator bool() const
. When called it checks the error flags. If either failbit or badbit are set then it returns false otherwise it returns true.
C++03
In this case stream has operator void*() const
. As this results in a pointer it can be used in a boolean context. When called it checks the error flags. If either failbit or badbit are set then it returns NULL which is equivalent to FALSE otherwise it returns a pointer to self (or something else valid though you should not use this fact)).
Usage
So you can use a stream in any context that would require a boolean test:
if (stream >> x)
{
}
while(stream)
{
/* do Stuff */
}
Note: It is bad idea to test the stream on the outside and then read/write to it inside the body of the conditional/loop statement. This is because the act of reading may make the stream bad. It is usually better to do the read as part of the test.
while(std::getline(steam, line))
{
// The read worked and line is valid.
}
Solution 2
Look from reference. The istream returned from getline
is converted to bool by implicit conversion to check success of operation. That conversion makes usage of if(mystream.getline(a,b))
into shorthand for if(!mystream.getline(a,b).fail())
.
Solution 3
It returns the stream itself. The stream can convert (through void*
) to bool
indicating its state. In this example, your while
loop will terminate when the stream's conversion to bool
goes "false", which happens when your stream enters an error state. In your code, it's most likely to occur when there was an attempt to read past the end of the file. In short, it'll read as much as there is, and then stop.
Solution 4
The function returns a reference to the stream object itself, which can be used either to chain further read operations:
myStream.getline(...).getline(...);
or, because streams are implicitly convertible to void *
s, in a loop or condition:
while (myStream.getline(...)) {
...
}
You can read more about this on the cplusplus.com website:
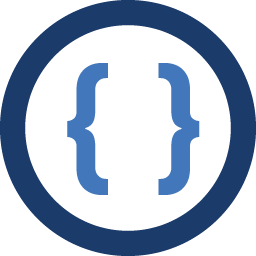
Admin
Updated on June 28, 2020Comments
-
Admin almost 4 years
What does the
istream::getline
method return?I am asking because I have seen that to loop through a file, it should be done like this:
while ( file.getline( char*, int ) ) { // handle input }
What is being returned?
-
Cameron over 13 years+1. It can convert to bool because it overloads the
operator bool()
(oroperator void*()
, not sure which) -
Lightness Races in Orbit over 13 years@Cameron: It's
operator void*()
. -
Admin over 13 yearsbut what if you want a
char*
instead of anstd::string
? that is the situation I am in. -
Nim over 13 years
std::string
has thec_str()
method which will return you what you require, if you need to modify the string, then there are methods ofstd::string
you can use to modify it. -
iheanyi almost 10 yearsYour link contains nothing that dovetails with your statement.
-
Öö Tiib almost 10 years@iheanyi Added clarification, perhaps the pages have changed over time.