Javascript Iframe innerHTML
Solution 1
I think this is what you want:
window.frames['iframe01'].document.body.innerHTML
EDIT:
I have it on good authority that this won't work in Chrome and Firefox although it works perfectly in IE, which is where I tested it. In retrospect, that was a big mistake
This will work:
window.frames[0].document.body.innerHTML
I understand that this isn't exactly what was asked but don't want to delete the answer because I think it has a place.
I like @ravz's jquery answer below.
Solution 2
Having something like the following would work.
<iframe id = "testframe" onload = populateIframe(this.id);></iframe>
// The following function should be inside a script tag
function populateIframe(id) {
var text = "This is a Test"
var iframe = document.getElementById(id);
var doc;
if(iframe.contentDocument) {
doc = iframe.contentDocument;
} else {
doc = iframe.contentWindow.document;
}
doc.body.innerHTML = text;
}
Solution 3
If you take a look at JQuery, you can do something like:
<iframe id="my_iframe" ...></iframe>
$('#my_iframe').contents().find('html').html();
This is assuming that your iframe parent and child reside on the same server, due to the Same Origin Policy in Javascript.
Solution 4
Conroy's answer was right. In the case you need only stuff from body tag, just use:
$('#my_iframe').contents().find('body').html();
Solution 5
You can use the contentDocument or contentWindow property for that purpose. Here is the sample code.
function gethtml() {
const x = document.getElementById("myframe")
const y = x.contentWindow || x.contentDocument
const z = y.document ? y.document : y
alert(z.body.innerHTML)
}
here, myframe
is the id of your iframe.
Note: You can't extract the content out of an iframe from a src outside you domain.
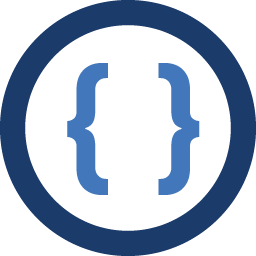
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Does anyone know how to get the HTML out of an IFRAME I have tried several different ways:
document.getElementById('iframe01').contentDocument.body.innerHTML document.frames['iframe01'].document.body.innerHTML document.getElementById('iframe01').contentWindow.document.body.innerHTML
etc
-
Prestaul over 15 yearsAnd if you want to be more unambiguous you could say: window.frames['iframe01'].document.body.innerHTML
-
Prestaul over 15 yearsYou are grabbing the innerHTML of the html element and not the body, and this is a pretty backwards method to do it. When there are native DOM properties for retrieving what you want (e.g. .body) you should avoid the overhead of a find call.
-
Olga about 12 yearsthis way does not and will not work in Firefox, because firefox does not support window.frames['frameId'] only window.frames[0] window.frames[1] etc
-
Miguel Alejandro Fuentes Lopez almost 11 yearsStop using a jQuery as an answer please!
-
leonneo over 10 yearsAgreed using JQuery as an answer is really annoying! Did the person who ask for that request for JQuery?
-
Michael Sazonov over 9 years@Prestaul there are people who try to think performance-wise, challenge-wise, program-wise. The others think jQuery is the solution to all problems...
-
Rusty Nail over 7 yearsBest Answer! Thanks! Don't forget: <head> <script src="ajax.googleapis.com/ajax/libs/jquery/3.1.1/…> </head>