Laravel validation: exists two column same row
Solution 1
I came across the same need today and I think I have a solution using the validation Rule class: Rule example.
Here is my scenario: I have an email verifications table and I want to ensure that a passed machine code and activation code exist on the same row.
Be sure to include use Illuminate\Validation\Rule;
$activationCode = $request->activation_code;
$rules = [
'mc' => [
'required',
Rule::exists('email_verifications', 'machineCode')
->where(function ($query) use ($activationCode) {
$query->where('activationCode', $activationCode);
}),
],
'activation_code' => 'required|integer|min:5',
'operating_system' => 'required|alpha_num|max:45'
];
The first argument in the exists method is the table and the second is the custom column name I'm using for the 'mc' field. I pass the second column I want to check using the 'use' keyword and then use that field in a a where clause.
Solution 2
You can simply use this:
'request_input' => exists:table,col,alternative_col_1,alternative_val_1,alternative_col_2,alternative_val_2, ...'
The SQL query is like below:
select count(*) as aggregate from `table` where `id` = [request_input] and `alternative_col1` = "alternative_val1" and `alternative_col2` = "alternative_val2"
Solution 3
You can achieve validating multipe columns by using Rule.
Include :
use Illuminate\Validation\Rule;
use Validator;
$AdminId = $request->AdminId;
$Validate = Validator::make($request->all(),[
'TaxId' => [ 'required', Rule::exists('tax','id')
->where(function ($query) use ($AdminId) {
$query->where('u_id', $AdminId);
})],
]);
if($Validate->fails())
{`Your ERROR HERE`}
else
{`SUCCESS`}
Here in my example I'm checking u_id and Id which is in same row and 2 columns.
Explaining : for example
My DB HAS 2 ROWS IN tax TABLE
id = 1 , u_id = 1, tax = "GST" , value -> 8
id = 2 , u_id = 2, tax = "GST" , value -> 5
1st scenario if the input id = 2 and u_id = 1 the validation must fail because this row belongs to u_id = 2.
2nd scenario if the input id = 2 and u_id = 2 the validation must success
Solution 4
i managed to solve it using the most simple method
- for
numero_de_somme
'numero_de_somme' => 'unique:personnels,numero_de_somme|exists:fonctionnaire,num_somme,cin,'.Request::get('cin'),
- for
cin
'cin' => 'unique:personnels,cin|exists:fonctionnaire,cin',
PS . don't forget to call use Illuminate\Http\Request;
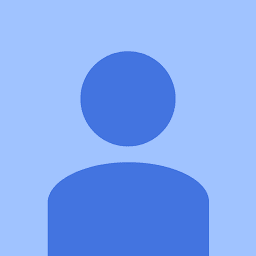
Miloud BAKTETE
Updated on June 13, 2022Comments
-
Miloud BAKTETE almost 2 years
Is there a way of referencing another field when specifying the exists validation rule in Laravel?
My request :
public function rules() { return [ 'numero_de_somme' => 'unique:personnels,numero_de_somme|exists:fonctionnaire,num_somme', 'cin' => 'unique:personnels,cin|exists:fonctionnaire,cin', ]; }
in my validation rules I want to be able to make sure that:
num_somme
exists within thefonctionnaire
tablecin
exists within the fonctionnaire table andcin
input must be on the same row of thenum_somme
num_somme : 12345 cin : s89745
num_somme : 78945 cin : U10125
Explaining : for example
- 1st scenario if the input
num_somme
= 12345 andcin
= U10125 the validation must fail - 2nd scenario if the input
num_somme
= 12345 andcin
= s89745 the validation must success
I hope this makes sense.
Thank you
-
Jacobo about 5 yearsWith num_somme on the exista rule you’re referring again to numero_de_somme?