listing Outlook emails by specific date in Python
Solution 1
Ive completed the code. Thanks for help.
`import sys, win32com.client, datetime
# Connect with MS Outlook - must be open.
outlook = win32com.client.Dispatch("Outlook.Application").GetNamespace
("MAPI")
# connect to Sent Items
s = outlook.GetDefaultFolder(5).Items # "5" refers to the sent item of a
folder
#s.Sort("s", true)
# Get yesterdays date for the purpose of getting emails from this date
d = (datetime.date.today() - datetime.timedelta (days=1)).strftime("%d-%m-%
y")
# get the email/s
msg = s.GetLast()
# Loop through emails
while msg:
# Get email date
date = msg.SentOn.strftime("%d-%m-%y")
# Get Subject Line of email
sjl = msg.Subject
# Set the critera for whats wanted
if d == date and msg.Subject.startswith("xx") or msg.Subject.startswith
("yy"):
print("Subject: " + sjl + " Date : ", date)
msg = s.GetPrevious() `
This works. However if no message according to the constraint if found, it doesnt exit. Ive tried break which just finds one message and not all, Im wondering if and how to do an exception? or if i try a else d != date it doenst work either (it will not find anything). I cant see that a For loop will work using a date with a msg(string). I not sure -- biginner here :) ??
Solution 2
The outlook API has a method, Items.Find
, for searching the contents of .Items
. If this is the extent of what you want to do, that's probably how you should do it.
Right now it seems like your if statement is checking whether set of emails is equal to yesterday.
Microsoft's documentation says .Items
is returning a collection of emails which you first must iterate through using a few different methods including Items.GetNext
or by referencing a specific index with Items.Item
.
You can then take the current email and access the .SentOn
property.
currentMessage = sent.GetFirst()
while currentMessage:
if currentMessage.SentOn == y:
sjl = currentMessage.Subject
print(sjl)
currentMessage = sent.GetNext()
This should iterate through all messages in the sent folder until sent.GetNext()
has no more messages to return. You will have to make sure y
is the same formatting as what .SentOn
returns.
If you don't want to iterate through every message, you could probably also nest two loops that goes back in messages until it gets to yesterday, iterates until it is no longer within "yesterday", and then breaks.
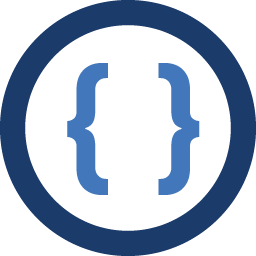
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm using Python 3. I'm trying to extract (list / print show) outlook emails by date.
I was trying a loop.. maybe WHILE or IF statement.
Can it be done since ones a string and the other is a date. Please concide what I've got so far: Thanks.
1. import win32com.client, datetime 2. 3. # Connect with MS Outlook - must be open. 4. outlook = win32com.client.Dispatch("Outlook.Application").GetNamespace("MAPI") 5. # connect to Sent Items 6. sent = outlook.GetDefaultFolder(5).Items # "5" refers to the sent item of a folder 7. 8. # Get yesterdays date 9. y = (datetime.date.today () - datetime.timedelta (days=1)) 10. # Get emails by selected date 11. if sent == y: 12. msg = sent.GetLast() 13. # get Subject line 14. sjl = msg.subject 14. # print it out 15. print (sjl)