Mailer error missing template
Solution 1
Try adding layout
class UserMailer < ActionMailer::Base
default from: "[email protected]"
layout "mailer"
def mailer(user)
@user = user
mail(to: @user.email, subject: 'Test')
end
end
Solution 2
Could you publish a minimalist Github repository reproducing your error?
You may try to generate your mailer in order to check you are not forgetting something:
bundle exec rails generate mailer mailer_classname mailer_viewname
In your case:
bundle exec rails generate mailer user_mailer mailer
Solution 3
class UserMailer < ActionMailer::Base
default from: "[email protected]"
layout "mailer"
def mailer(user)
@user = user
mail(to: @user.email, subject: 'Test')
end
end
Add a html layout under layout#
mailer.html.erb
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<style>
/* Email styles need to be inline */
</style>
</head>
<body>
<%= yield %>
</body>
</html>
Add text layout under layout #
mailer.text.erb
<%= yield %>
Use preview to check your email based on your test framework.
Solution 4
I had the same problem. Restarting sidekiq solved it for me.
Solution 5
It's likely that Rails just isn't finding your newly created files. If you have the spring
gem running, that could be the issue. (More on spring: https://github.com/rails/spring)
To setup terminal commands and stop running spring
:
$ bundle exec spring binstub --all
$ bin/spring stop
I tried this, then re-running my app with rails s
. That didn't work so then try closing the terminal completely and rebooting your computer. Run rails s
and try testing with rails c
in a second tab:
mail = UserMailer.mailer(User.last)
That finally worked for me, hopefully it'll help some of you!
(Some of these steps may be redundant.)
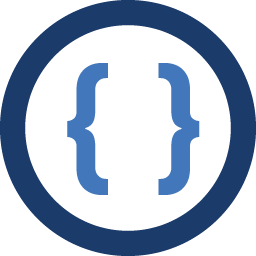
Admin
Updated on June 02, 2022Comments
-
Admin almost 2 years
Hello,
I have problem with ActionMailer, when I try to execute action:
rake send_email
I get a error:
rake aborted! ActionView::MissingTemplate: Missing template user_mailer/mailer with "mailer". Searched in: * "user_mailer"
Here's my:
mailers/user_mailer.rb
class UserMailer < ActionMailer::Base default from: "[email protected]" def mailer(user) @user = user mail(to: @user.email, subject: 'Test') end end
views/user_mailer/mailer.html.erb
<!DOCTYPE html> <html> <head> <meta content='text/html; charset=UTF-8' http-equiv='Content-Type' /> </head> <body> <p> Sample mail. </p> </body> </html>
views/user_mailer/mailer.text.erb
Sample mail.
lib/tasks/emails_task.rake
desc 'send email' task send_email: :environment do UserMailer.mailer(User.last).deliver! end
config/environments/development.rb
# I recommend using this line to show error config.action_mailer.raise_delivery_errors = true # ActionMailer Config config.action_mailer.delivery_method = :letter_opener # config.action_mailer.default_url_options = { :host => 'localhost:3000' } # config.action_mailer.delivery_method = :smtp # SMTP settings for gmail config.action_mailer.smtp_settings = { :address => "smtp.gmail.com", :port => 587, :user_name => ENV['gmail_username'], :password => ENV['gmail_password'], :authentication => "plain", :enable_starttls_auto => true } # Send email in development mode? config.action_mailer.perform_deliveries = true
I searched for the solution on stackoverflow and I tried many of the answers for the similar problem but unfortunately none of them worked for me.
I found solution, when I add body to mailer method like:
def mailer(user) @user = user mail(to: @user.email, subject: 'Test', body: 'something') end
Then it does work but I would like to have a body in separate files and make it more complex with user name and other things.
If someone have a idea how to solve this problem then I would be very thankful :)
-
Christian Fazzini over 7 yearsCan you be specific? What method exactly?
-
montrealmike almost 6 years@ChristianFazzini
def mailer(user)