Material-ui v1 Input focus style override
12,254
The best way to accomplish that is to override the focused
style exposed by the Input
component, but using classes instead of class names.
To do so, you should first create a CSS style class specifically for the focused input:
const styles = theme => ({
input: {
width: '20%',
borderRadius: 4,
backgroundColor: 'white',
border: '1px solid #ced4da',
fontSize: 20,
},
// Separate this part into it's own CSS class
inputFocused: {
width: '40%',
borderColor: '#80bdff',
boxShadow: '0 0 0 0.2rem rgba(0,123,255,.25)',
backgroundColor: "#00FF00",
},
});
Then override the focused
style on the Input
like this:
<Input
className={classes.input}
classes={{ focused: classes.inputFocused}}
/>
When you join that all together, a full working example would look like this:
import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from 'material-ui/styles';
import Input from 'material-ui/Input';
const styles = theme => ({
input: {
width: '20%',
borderRadius: 4,
backgroundColor: 'white',
border: '1px solid #ced4da',
fontSize: 20,
},
// Separate this part into it's own CSS class
inputFocused: {
width: '40%',
borderColor: '#80bdff',
boxShadow: '0 0 0 0.2rem rgba(0,123,255,.25)',
backgroundColor: "#00FF00",
},
});
function Inputs(props) {
const { classes } = props;
return (
<div className={classes.container}>
<Input
className={classes.input}
classes={{ focused: classes.inputFocused}}
/>
</div>
);
}
Inputs.propTypes = {
classes: PropTypes.object.isRequired,
};
export default withStyles(styles)(Inputs);
You can read more about overriding a component's styles with classes here.
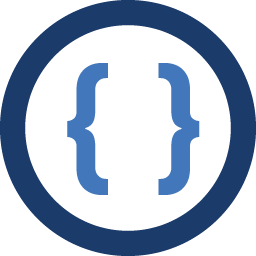
Author by
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I'm trying to override the styling of the Input component when it is on focus via class name overide.
I have tried the following:
const style = theme => ({ input: { width: '20%', borderRadius: 4, backgroundColor: 'white', border: '1px solid #ced4da', fontSize: 20, '&:focus': { width: '40%', borderColor: '#80bdff', boxShadow: '0 0 0 0.2rem rgba(0,123,255,.25)', }, } }); class test extends Component { // UI render() { const {classes} = this.props return ( <AppBar position="static"> <Toolbar> <Input className={classes.input} /> </Toolbar> </AppBar> ); } } export default withStyles(style)(test);
Thank you
-
Pablo Rincon about 5 yearsSilly question, but that is the difference between className and classes used within <Input> ?
-
nrmad over 2 yearsclasses can target specific elements used in building the component