Missing partial modifier on declaration ..another partial declaration of this type exists". I'm a beginner and just following the book
Solution 1
In another place you declared a class with the same name (Form1
) and there it's declared with the partial
modifier.
If you're splitting your class into two different files (for example this file for UI layout and another file for logic) then simply add the partial
modifier to the declaration:
public partial class Form1
{
}
Solution 2
When you create a new form, it is actually created in two separate places. one place the class is declared is where all the plumbing is being implemented, and the file that you pasted here, to write all the functionality. for the sake of making this possible, c# has a partial class feature, if in some place of the assembly a class is defined with a partial modifier, then one can define multiple declarations of the class, as long as it has that modifier.
Solution 3
In my case this error occurred when I was using a web form application in asp.net
and it was because in my global.asax
markup, I had replace CodeBehind
Attribute with CodeFile
. Changing it back fixed the problem.
Solution 4
Just in case someone stumbles into this like me... I have been switching back and forth between Visual Studio 2013 for Web and Visual Studio 2015 RC to play with my MVC solutions.
If you attempt to open an MVC4 solution in 2015 RC it does some automagic which can cause this error to come up next time you open that same solution in 2013 for Web.
I ended up rolling back to a previous SVN revision and only using 2013 for Web and my code built fine just like it had for years. I know this isn't the proper fix but this is what caused this to 'pop up' out of nowhere in my instance.
Solution 5
TL;DR: caused by vestiges of classes that were renamed by VS2013 but that VS2013 didn't properly rename everywhere, leaving instances of the old name hanging about in undisclosed places.
DETAIL: I had the exact same error in VS2013, in my case initially I added a new class "Employee" but then realized it should have been AddEmployee so I right-clicked and changed the name and it 'appeared' to change it in all the right places.
Quite a while later I tried to add an Employee class and then got the error. After visiting here and not finding a fix, I searched 'entire solution' for other instances of Employee and that turned up a couple of fails from when I had previously renamed Employee to AddEmployee:
AddEmployee.aspx.cs: public partial class Employee : System.Web.UI.Page
AddEmployee.aspx.designer.cs: public partial class Employee {
Updated those two to AddEmployee and the error went away and compiled and ran as it should..
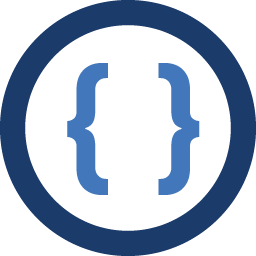
Admin
Updated on October 20, 2020Comments
-
Admin over 3 years
I'm a beginner and I'm doing an exercise following a book. Below is my code and i got this
"Missing partial modifier on declaration of type 'Windowsform.Form1'; another partial declaration of this type exists"
.What should i do? My code is as follows:
using System; using System.Windows.Forms; namespace Windowsform { public class Form1 : Form { private TextBox txtEnter; private Label lblDisplay; private Button btnOk; public Form1() { this.txtEnter = new TextBox(); this.lblDisplay = new Label(); this.btnOk = new Button(); this.Text = "My Hellowin app!"; //txtEnter this.txtEnter.Location = new System.Drawing.Point(16, 32); this.txtEnter.Size = new System.Drawing.Size(264, 20); //lblDisplay this.lblDisplay.Location = new System.Drawing.Point(16, 72); this.lblDisplay.Size = new System.Drawing.Size(264, 128); //btnOK this.btnOk.Location = new System.Drawing.Point(88, 224); this.btnOk.Text = "OK"; this.btnOk.Click += new System.EventHandler(this.btnOK_Click); //MyForm this.Controls.AddRange(new Control[] { this.txtEnter, this.lblDisplay, this.btnOk}); } static void Main() { Application.Run(new Form1()); } private void btnOK_Click(object sender, System.EventArgs e) { lblDisplay.Text = txtEnter.Text + "\n" + lblDisplay.Text; } } }
-
Adriano Repetti over 11 years@user1742237 I guess you did change the default generated Program class to include the Form1 declaration but usually the template for a WinForms application includes a generated Form1 class with an empty Form. Simply delete it.
-
Don Cheadle about 8 yearsI had to scroll this far to find an actual straight-forward, code-oriented answer? On StackOverflow? ...
-
AKS about 5 yearsExactly same happen with me, switching but between VS 2015 and VS2019 causes this.