Moving specific file types with Python
Solution 1
You're trying to move the whole source folder, you need to specify a file path
import os
import shutil
sourcepath='C:/Users/kevinconnell/Desktop/Test_Folder/'
sourcefiles = os.listdir(sourcepath)
destinationpath = 'C:/Users/kevinconnell/Desktop/Test_Folder/Archive'
for file in sourcefiles:
if file.endswith('.png'):
shutil.move(os.path.join(sourcepath,file), os.path.join(destinationpath,file))
Solution 2
Another option is using glob module, which let's you specify file mask and retrieve list of desired files. It should be as simple as
import glob
import shutil
# I prefer to set path and mask as variables, but of course you can use values
# inside glob() and move()
source_files='/Users/kevinconnell/Desktop/Test_Folder/*.png'
target_folder='/Users/kevinconnell/Dekstop/Test_Folder/Archive'
# retrieve file list
filelist=glob.glob(source_files)
for single_file in filelist:
# move file with full paths as shutil.move() parameters
shutil.move(single_file,target_folder)
Nevertheless, if you're using glob or os.listdir, remember to set full paths for source file and target.
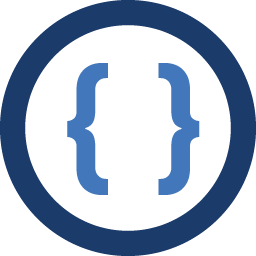
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I know this is going to be frustratingly easy for many of you. I am just beginning to learn Python and need help with some basic file handling.
I take a lot of screenshots, which end up on my desktop (as this is the default setting). I am aware I can change the screenshot setting to save it somewhere else automatically. However, I think this program will be a good way to teach me how to sort files. I would like to use python to automatically sort through all the files on my desktop, identify those that end with .png (the default file type for screenshots), and simply move it to a folder I've named "Archive".
This is what I've got so far:
import os import shutil source = os.listdir('/Users/kevinconnell/Desktop/Test_Folder/') destination = 'Archive' for files in source: if files.endswith('.png'): shutil.move(source, destination)
I've played around with it plenty to no avail. In this latest version, I am encountering the following error when I run the program:
Traceback (most recent call last): File "pngmove_2.0.py", line 23, in shutil.move(source, destination) File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/shutil.py", line 290, in move TypeError: coercing to Unicode: need string or buffer, list found
I am under the impression I have a sort of issue with the proper convention/syntax necessary for the source and destination. However, I've thus far been unable to find much help on how to fix it. I used os.path.abspath() to determine the file path you see above.
Thanks in advance for any help in preserving my sanity.
LATEST UPDATE
I believe I am very close to getting to the bottom of this. I'm sure if I continue to play around with it I'll figure it out. Just so everyone that's been helping me is updated...
This is the current code I'm working with:
import os import shutil sourcepath ='/Users/kevinconnell/Desktop/' source = os.listdir(sourcepath) destinationpath = '/Users/kevinconnell/Desktop/' for files in source: if files.endswith('.png'): shutil.move(os.path.join(sourcepath,'Test_Folder'), os.path.join(destinationpath,'Archive'))
This works for renaming my 'Test_Folder' folder to 'Archive'. However, it moves all the files in the folder, instead of moving the files that end with '.png'.
-
AMacK almost 10 yearsI'd also recommend using os.path.join instead of +
-
sundar nataraj almost 10 years@AMacK i have showed him what is wrong, please update my answer. so that i can learn better
-
sundar nataraj almost 10 years@nivackz i said where did error caused since u r sending source which is list of files but shutill.move need single file. that what i meant. he said he prefer using os.join.path to join the sourcepath+file name. Please there is nothing against u
-
AMacK almost 10 yearsSorry it took me a bit to get back. I think you covered everything I noticed, although there's a missing "/" at the beginning of destination path.
-
sundar nataraj almost 10 years@AMacK thanks for ur idea. Really os.path.join is must. some times v forget giving slashes properly or it takes as special character
-
Gabriel M almost 10 yearsAfter your update - glob handles your filemask problems easily.
-
birdmw almost 7 yearsdid you mean for single_file in filelist?