Navigation Drawer with Custom Button
Solution 1
Use GlobalKey and show the drawer by calling myKey.currentState.openDrawer()
In demo, I also open drawer when click button
full code
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: ListenToDrawerEvent(),
);
}
}
class ListenToDrawerEvent extends StatefulWidget {
@override
ListenToDrawerEventState createState() {
return new ListenToDrawerEventState();
}
}
class ListenToDrawerEventState extends State<ListenToDrawerEvent> {
GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
static final List<String> _listViewData = [
"Inducesmile.com",
"Flutter Dev",
"Android Dev",
"iOS Dev!",
"React Native Dev!",
"React Dev!",
"express Dev!",
"Laravel Dev!",
"Angular Dev!",
];
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: Text("Listen to Drawer Open / Close Example"),
leading: IconButton(
icon: Icon(Icons.menu),
onPressed: () {
_scaffoldKey.currentState.openDrawer();
},
),
),
drawer: Drawer(
child: ListView(
padding: EdgeInsets.all(10.0),
children: _listViewData
.map((data) => ListTile(
title: Text(data),
))
.toList(),
),
),
body: Column(
children: <Widget>[
Center(
child: Text('Main Body'),
),
RaisedButton(
padding: const EdgeInsets.all(8.0),
textColor: Colors.white,
color: Colors.blue,
onPressed: () {_scaffoldKey.currentState.openDrawer();},
child: new Text("open drawer"),
)
],
),
);
}
}
Solution 2
AppBar(
leading: Builder(
builder: (BuildContext context) {
return IconButton(
icon: const Icon(Icons.menu),
onPressed: () { Scaffold.of(context).openDrawer(); },
tooltip: MaterialLocalizations.of(context).openAppDrawerTooltip,
);
},
),
)
See also:
- https://api.flutter.dev/flutter/material/AppBar/leading.html
- https://www.youtube.com/watch?v=EcAwFpC9S8s
Solution 3
if (_scaffoldKey.currentState.isDrawerOpen)
_scaffoldKey.currentState.openEndDrawer();
else {
_scaffoldKey.currentState.openDrawer();
}
final GlobalKey<ScaffoldState> _scaffoldKey = new GlobalKey<ScaffoldState>();
You can manipulate depend on which side you have your drawer!!!!
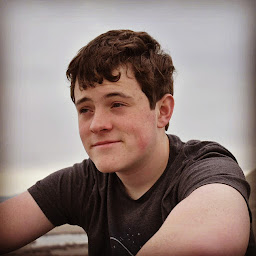
Alex Collette
I am a software engineer at Evoca TV in Boise Idaho, working to launch a nextGen TV service based on ATSC 3.0. I primarily write code in Javascript and Dart, as well as working with AWS and related services. I am also a part-time student at Boise State University, studying Computer Science.
Updated on December 14, 2022Comments
-
Alex Collette over 1 year
I am currently trying to set up a search bar for my app that looks like the photo below. Currently I have a widget that works well as the search bar, but I am trying to implement a navigation drawer that uses that button. Is there a way to tie a navigation drawer to it? Do I need to recreate this widget in an appBar?
I am not sure what the best way to accomplish this is, and I would love some suggestions about how to proceed!
Thanks!
-
Alex Collette over 4 yearsThank you so much! This worked like a charm! I also appreciate how detailed your response was:)
-
K_Chandio about 2 years@chunhunghan can you please tell me, I am not using drawer and appbar in my app, how can i open drawer on clicking specific button.