PHP String to Float
Solution 1
$rootbeer = (float) $InvoicedUnits;
Should do it for you. Check out Type-Juggling. You should also read String conversion to Numbers.
Solution 2
You want the non-locale-aware floatval
function:
float floatval ( mixed $var ) - Gets the float value of a string.
Example:
$string = '122.34343The';
$float = floatval($string);
echo $float; // 122.34343
Solution 3
Well, if user write 1,00,000 then floatvar will show error. So -
floatval(preg_replace("/[^-0-9\.]/","",$input));
This is much more reliable.
Usage :
$input = '1,03,24,23,434,500.6798633 this';
echo floatval(preg_replace("/[^-0-9\.]/","",$input));
Solution 4
Dealing with markup in floats is a non trivial task. In the English/American notation you format one thousand plus 46*10-2
:
1,000.46
But in Germany you would change comma and point:
1.000,46
This makes it really hard guessing the right number in multi-language applications.
I strongly suggest using Zend_Measure
of the Zend Framework for this task. This component will parse the string to a float by the users language.
Solution 5
you can follow this link to know more about How to convert a string/number into number/float/decimal in PHP. HERE IS WHAT THIS LINK SAYS...
Method 1: Using number_format() Function. The number_format() function is used to convert a string into a number. It returns the formatted number on success otherwise it gives E_WARNING on failure.
$num = "1000.314";
//Convert string in number using
//number_format(), function
echo number_format($num), "\n";
//Convert string in number using
//number_format(), function
echo number_format($num, 2);
Method 2: Using type casting: Typecasting can directly convert a string into a float, double, or integer primitive type. This is the best way to convert a string into a number without any function.
// Number in string format
$num = "1000.314";
// Type cast using int
echo (int)$num, "\n";
// Type cast using float
echo (float)$num, "\n";
// Type cast using double
echo (double)$num;
Method 3: Using intval() and floatval() Function. The intval() and floatval() functions can also be used to convert the string into its corresponding integer and float values respectively.
// Number in string format
$num = "1000.314";
// intval() function to convert
// string into integer
echo intval($num), "\n";
// floatval() function to convert
// string to float
echo floatval($num);
Method 4: By adding 0 or by performing mathematical operations. The string number can also be converted into an integer or float by adding 0 with the string. In PHP, performing mathematical operations, the string is converted to an integer or float implicitly.
// Number into string format
$num = "1000.314";
// Performing mathematical operation
// to implicitly type conversion
echo $num + 0, "\n";
// Performing mathematical operation
// to implicitly type conversion
echo $num + 0.0, "\n";
// Performing mathematical operation
// to implicitly type conversion
echo $num + 0.1;
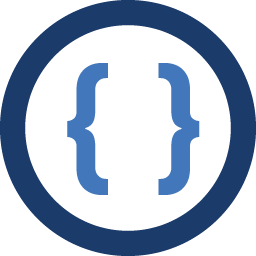
Admin
Updated on June 10, 2020Comments
-
Admin almost 4 years
I am not familiar with PHP at all and had a quick question.
I have 2 variables
pricePerUnit
andInvoicedUnits
. Here's the code that is setting these to values:$InvoicedUnits = ((string) $InvoiceLineItem->InvoicedUnits); $pricePerUnit = ((string) $InvoiceLineItem->PricePerUnit);
If I output this, I get the correct values. Lets say
5000
invoiced units and1.00
for price.Now, I need to show the total amount spent. When I multiply these two together it doesn't work (as expected, these are strings).
But I have no clue how to parse/cast/convert variables in PHP.
What should I do?
-
Talvi Watia almost 14 yearsIts the most simple method.. but occasionally I have run into issues doing this with certain database configs and
VARCHAR>11
in length.. haven't narrowed down exactly why yet. -
ontananza almost 12 yearsInstead of ereg use preg
$string=preg_replace("/[^0-9\.\-]/","",$string);
-
HADI almost 12 yearsYes, ereg, This function has been DEPRECATED. So need to use preg instead ereg :)
-
zamnuts over 10 yearsNowadays (+3 years), one could use NumberFormatter as of PHP 5.3 with the
intl
extension. -
Tobias P. over 10 yearsPHP 5.3 had been available when i answered the question, but until your comment i was not aware of this single function as there are dozens of libraries doing something similar. But they won't have such a good coverage of locales as the intl extension has. Thanks, i'll try to remember it.
-
Dr. Gianluigi Zane Zanettini over 8 yearskudos for mentioning the locale-awareness. I just spent 1,5h tracking down an issue caused by different locales causing
(float)
to convert on the first server to "," and on the second to "," -
Admin over 7 years^worth noting that (float) removes 0 from string if it's the first digit
-
Hafenkranich about 7 yearsany comments on why this is bad / where does it fail?
-
Hafenkranich almost 7 yearsor is there a likewise flexible way which is faster?
-
Leif over 6 yearsIt uses the e flag, which is "no longer supported". That's my guess.
-
CodeJunkie over 4 yearsAlso note that "11,000.00" will output 11 instead of the expected 11000.00
-
Uriahs Victor over 3 yearsAdding the output that would be received in each of those cases as a comment would help a ton without us having to test out each scenario
-
Mujahid Bhoraniya about 3 years@HADI Nice working for me. very appriciate dear
-
mickmackusa over 2 yearsA
.
inside of a character class does not need to be escaped.