Problems while using Flutter Provider package
992
Try to add listen: false
to the provider call :
Provider.of<Data>(context, listen: false).toggle();
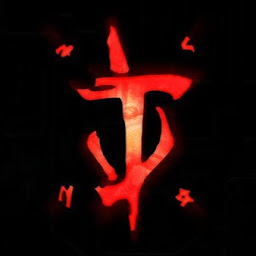
Author by
Lakshay Dutta
I like to be informative about everything so there is rarely any technology or tool that I don't try, albeit for one day only.I also like PC gaming and creating vector graphics
Updated on December 20, 2022Comments
-
Lakshay Dutta over 1 year
I am creating a very simple app to practice flutter provider package. The app has a bulb which on click , should change it's background and the screen's background , using provider. But this doesnt seem to work. TBH this is a lot confusing
import 'package:flutter/material.dart'; import 'package:provider/provider.dart'; void main() => runApp(MainApp()); class Data extends ChangeNotifier{ bool isOn = false; void toggle(){ this.isOn = !this.isOn; notifyListeners(); print("new value is $isOn"); } } class MainApp extends StatelessWidget { @override Widget build(BuildContext context) { return ChangeNotifierProvider<Data>( create: (context) => Data(), child: MaterialApp( home: Home(), ), ); } } class Home extends StatefulWidget { @override _HomeState createState() => _HomeState(); } class _HomeState extends State<Home> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(), backgroundColor: Provider.of<Data>(context).isOn ? Colors.yellow[100] : Colors.black, body: Center( child: Column( children: <Widget>[ Stick(), Bulb(), ], ), ), ); } } class Stick extends StatelessWidget { @override Widget build(BuildContext context) { return Container( height: 150, width: 40, color: Colors.brown, ); } } class Bulb extends StatefulWidget { @override _BulbState createState() => _BulbState(); } class _BulbState extends State<Bulb> { @override Widget build(BuildContext context) { return Container( height: 200, width: 250, decoration: BoxDecoration( borderRadius: BorderRadius.only( topLeft: Radius.circular(100), topRight: Radius.circular(100), bottomLeft: Radius.circular(30), bottomRight: Radius.circular(30)), color: Provider.of<Data>(context).isOn ? Colors.yellow : Colors.white, ), child: GestureDetector( onTap: (){ Provider.of<Data>(context).toggle(); setState(() { }); }, ), ); } }
The tree structure has a main app which houses a Home app , which houses 2 other widgets , a stick and a bulb inside a container. I am trying to update the background of bulb and the Home widget when the bulb is clicked. Bulb has a gesture detector Any kind of hint or help appreciated
-
Lakshay Dutta about 4 yearsIt worked ! Please explain how did that work ! Also do point out if there is anything wrong with the way I am using provider
-
MickaelHrndz about 4 yearsI don't know exactly why, but this is the doc on it : If [listen] is true, later value changes will trigger a new [State.build] to widgets, and [State.didChangeDependencies] for [StatefulWidget].
-
Lakshay Dutta almost 4 yearsFor anyone still looking for other ways to use provider, try Consumer<T> widget. it is better than calling Provider.of( ) each time