Python: Random numbers into a list
Solution 1
You could use random.sample
to generate the list with one call:
import random
my_randoms = random.sample(range(100), 10)
That generates numbers in the (inclusive) range from 0 to 99. If you want 1 to 100, you could use this (thanks to @martineau for pointing out my convoluted solution):
my_randoms = random.sample(range(1, 101), 10)
Solution 2
import random
my_randoms = [random.randrange(1, 101, 1) for _ in range(10)]
Solution 3
Fix the indentation of the print
statement
import random
my_randoms=[]
for i in range (10):
my_randoms.append(random.randrange(1,101,1))
print (my_randoms)
Solution 4
This is way late but in-case someone finds this helpful.
You could use list comprehension.
rand = [random.randint(0, 100) for x in range(1, 11)]
print(rand)
Output:
[974, 440, 305, 102, 822, 128, 205, 362, 948, 751]
Cheers!
Solution 5
Here I use the sample
method to generate 10 random numbers between 0 and 100.
Note: I'm using Python 3's range
function (not xrange
).
import random
print(random.sample(range(0, 100), 10))
The output is placed into a list:
[11, 72, 64, 65, 16, 94, 29, 79, 76, 27]
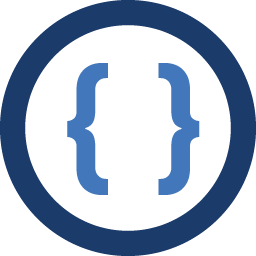
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
Create a 'list' called my_randoms of 10 random numbers between 0 and 100.
This is what I have so far:
import random my_randoms=[] for i in range (10): my_randoms.append(random.randrange(1, 101, 1)) print (my_randoms)
Unfortunately Python's output is this:
[34] [34, 30] [34, 30, 75] [34, 30, 75, 27] [34, 30, 75, 27, 8] [34, 30, 75, 27, 8, 58] [34, 30, 75, 27, 8, 58, 10] [34, 30, 75, 27, 8, 58, 10, 1] [34, 30, 75, 27, 8, 58, 10, 1, 59] [34, 30, 75, 27, 8, 58, 10, 1, 59, 25]
It generates the 10 numbers like I ask it to, but it generates it one at a time. What am I doing wrong?
-
Jon Clements almost 11 yearsSample won't include duplicate values, which may or may not matter in this use case, but worth noting
-
martineau almost 11 yearsFor values in the range of 1..100 it would be simpler to use
random.sample(xrange(1, 101), 10)
than a list comprehension. -
shakram02 about 7 yearsfor python3
my_randoms = random.sample(range(100), 10)
-
yakobom almost 7 yearsThanks for your answer. A short explanation regarding what he was doing wrong, as requested, would be great.
-
Peter Mortensen over 5 yearsFor other beginners (future readers), perhaps expand with an explanation regarding indentation error?
-
Cbhihe about 5 yearsThis is actually the only answer that addresses OP's query. Others are good of course, but they just improve/provide alternative on syntax, or methods, etc. OP's problem was just indentation, guys !
-
Cbhihe about 5 yearsActually, between 0 and 99 without replacement.
-
thanos.a over 4 yearsI get an error that 'xrange' is not defined. Do I miss any libraries?
-
robertklep over 4 years@thanos.a
xrange
doesn't exist anymore in Python 3, but you can userange
instead: geeksforgeeks.org/range-vs-xrange-python