Python - Write Co-ordinates into array
Solution 1
You can insert a tuple (X,Y) containing the x,y coordinates into a list.
>>>l=[]
>>>coords = tuple([2,4])
>>>l.append(coords)
>>>l
[(2,3)]
Solution 2
You can use the tuple
and list
types:
my_coords = [(1, 2), (3, 4)]
my_coords.append((5, 6))
for x, y in my_coords:
print(x**2 + y**2)
Some examples for coordinates
Points inside unit circle
my_coords = [(0.5, 0.5), (2, 2), (5, 5)]
result = []
for x, y in my_coords:
if x**2 + y**2 <= 1:
result.append((x, y))
Generating a circle
from math import sin, cos, radians
result = []
for i in range(360):
x = cos(radians(i))
y = -sin(radians(i))
result.append((x, y))
Solution 3
If you know the size of the data in advance, use a numpy array.
import numpy
arr = numpy.array([ [x,y] for x,y in get_coords() ])
If you need to append data on-the-fly, use a list of tuples.
l = []
for x,y in get_coords():
l.append((x,y))
Solution 4
A tuple is probably sufficient for simple tasks, however you could also create a Coordinate
class for this purpose.
class Coordinate(object):
def __init__(self, x, y):
self.x = x
self.y = y
def __repr__(self):
return 'Coordinate({0.x:d}, {0.y:d})'.format(self)
>>> coord = Coordinate(0, 0)
Coordinate(0, 0)
>>> coord.x = 1
>>> coord.y = 2
>>> coord
Coordinate(1, 2)
>>> coord.x
1
>>> coord.y
2
Notes
Python 2.5 compatibility
Use the following __repr__
method:
def __repr__(self):
return 'Coordinate(%d, %d)'.format(self)
Floating point coordinates
Floating point coordinates will be stored as is, but the __repr__
method will round them. Simply replace :d
with :.2f
(the same for %
), where 2
is the number of decimal points to display.
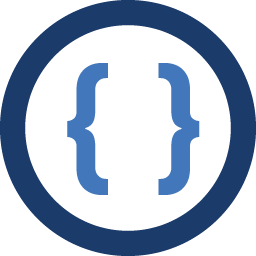
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I was wondering how best to write x,y co-ordinates into an array?
I also don't know how big the array will become...
Or perhaps, is there another data structure that would be easier to use?
Thanks for your help.