QC : How to Find a test in QC by test case id using OTA API in C#
11,135
Solution 1
Assuming you have an open connection and know a test with that ID exists (e.g. results from a previous query), you can do the following:
int testId = 1234;
TestFactory tf = connection.TestFactory;
Test t = tf[id];
The call to the TestFactory indexer will raise a COMException with "Item does not exist." if a test with that ID does not exist.
If you don't know for sure if a test with that ID exists, you can do the following:
int testId = 1234;
TestFactory tf = connection.TestFactory;
TDFilter filter = tf.Filter;
filter["TS_TEST_ID"] = id.ToString();
List tests = tf.NewList(filter.Text); // List collection is the ALM List, not .NET BCL List.
Test t = (tests.Count > 0) ? tests[1] : null; // ALM List collection indexes are 1-based, and test ID is guaranteed to be unique and only return 1 result if it exists.
Solution 2
You can create filter on Conection. Example below searches test by it's name, but if you'll change "TS_TEST_NAME" on "TS_TEST_ID" you'll be able to search using test ID.
public int findTestCase(TDAPIOLELib.TDConnection connection, string testCaseName)
{
TestFactory tstF = connection.TestFactory as TestFactory;
TDFilter testCaseFilter = tstF.Filter as TDFilter;
testCaseFilter["TS_TEST_NAME"] = testCaseName;
List testsList = tstF.NewList(testCaseFilter.Text);
if(testsList != null && testsList.Count == 1)
{
log.log("Test case " +testCaseName+ " was found ");
Test tmp = testsList[0] as Test;
return (int)tmp.ID;
}
return -1;
}
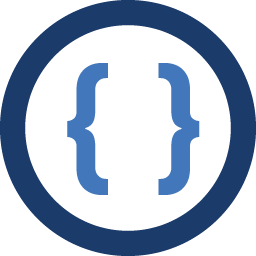
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I am using OTA API in C#.
How can I find a test in QC, if I am having test case ID. (Find Test by Test Case Id) Test may present under any folder in Test Plan.(i.e Under SUbject).