QFile won't open the file
24,907
Before opening the file, you can always check the existense:
QFile file("myfile.txt");
if (!file.exists()) {
// react
}
If file exists but does not open, you can get the error state and message:
QString errMsg;
QFileDevice::FileError err = QFileDevice::NoError;
if (!file.open(QIODevice::ReadOnly)) {
errMsg = file.errorString();
err = file.error();
}
And always: if the file was openend, then remember to close it. In your example you didn't:
file.close();
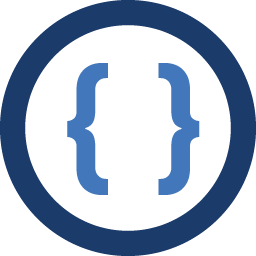
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a program that I basically stole from the Qt website to try to get a file to open. The program refuses to open anything I am confused as to why. I have looked for lots of documentation but found nothing can you please explain why it does not work.
#include "mainwindow.h" #include "ui_mainwindow.h" #include <QFile> #include <QTextStream> #include <QString> MainWindow::MainWindow(QWidget *parent) : QWidget(parent) { QFile file("C:/n.txt"); if (!file.open(QIODevice::ReadOnly | QIODevice::Text)) return; QTextStream in(&file); QString f=in.readLine(); lab =new QLabel("error",this); lab->setGeometry(100,100,100,100); lab->setText(f); }
-
Admin over 11 yearsThen it should be
QFile file("C:\\n.txt");
because backslashes need to be escaped. But / works fine in Windows so there is no need to change it. -
hyde over 11 yearsIndeed, when supported, using
/
is definitely preferred path separator in strings inside application. No reason to make porting any harder, and no reason to use char which needs escaping inside strings (with the potential to forget, and with bad luck just silently get a wrong char). -
Admin over 11 yearsFile is automatically closed when its destructor is called.
-
Manmohan Singh over 7 yearsDon't know if the op found answer to this question, but most of the times, this happens because of permission issues. If you're debugging your program, try opening QtCreator in administrator mode, and then debug. While running your program, open a cmd.exe window in admin mode and run the executable. Also, QFile works both ways: i.e. QFile("C:/n.txt") as well as QFile("C:\\n.txt") on windows, though the preferable way is to use forward slashes in file path.