Randomly shuffle rows in a large text file
Solution 1
You can use the shuf
command from GNU coreutils. The utility is pretty fast and would take less than a minute for shuffling a 1 GB file.
The command below might just work in your case because shuf
will read the complete input before opening the output file:
$ shuf -o File.txt < File.txt
Solution 2
Python one-liner:
python -c 'import sys, random; L = sys.stdin.readlines(); random.shuffle(L); print "".join(L),'
Reads all the lines from the standard input, shuffles them in-place, then prints them without adding an ending newline (notice the ,
from the end).
Solution 3
If like me you came here to look for an alternate to shuf
for macOS then use randomize-lines
.
Install randomize-lines
(homebrew) package, which has an rl
command which has similar functionality to shuf
.
brew install randomize-lines
Usage: rl [OPTION]... [FILE]...
Randomize the lines of a file (or stdin).
-c, --count=N select N lines from the file
-r, --reselect lines may be selected multiple times
-o, --output=FILE
send output to file
-d, --delimiter=DELIM
specify line delimiter (one character)
-0, --null set line delimiter to null character
(useful with find -print0)
-n, --line-number
print line number with output lines
-q, --quiet, --silent
do not output any errors or warnings
-h, --help display this help and exit
-V, --version output version information and exit
Solution 4
For OSX the binary is called gshuf
.
brew install coreutils
gshuf -o File.txt < File.txt
Related videos on Youtube
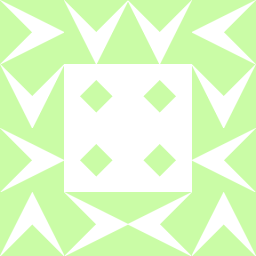
ddmichael
Updated on September 18, 2022Comments
-
ddmichael 9 months
I have a text file of ~1GB with about 6k rows (each row is very long) and I need to randomly shuffle its rows. Is it possible? Possibly with awk?
-
ddmichael about 9 yearsThanks, I forgot to mention I am on OSX, any equivalents?
-
brevno about 9 years@ddmichael Run
brew install coreutils
and use/usr/local/bin/gshuf
. -
Cristian Ciupitu about 9 yearsThat program is part of coreutils, as mentioned by Suraj Biyani.
-
Suraj Biyani about 9 years@ddmichael Alternatively for OS X you can use this Perl one liner. Got this one one of the old blogs. Did a quick test and found working.
cat myfile | perl -MList::Util=shuffle -e 'print shuffle(<STDIN>);'
I am note sure how fast would it run though