ReactJS connection with database
92,351
Lets take an example of a simple library application having table(books
) with fields book_name
and author
.
Lets see the Backend Code First(in Node Js)
const express = require('express');
const bodyParser = require('body-parser');
var connection = require('express-myconnection');
var mysql = require('mysql');
const app = express();
app.use(bodyParser.json());
app.use(
connection(mysql,{
host: 'localhost', //'localhost',
user: 'userEHX',
password : 'hMmx56FN4GHpMXOl',
port : 3306, //port mysql
database:'sampledb'
},'pool')); //or single
app.post('/add_book',(req,res)=>{
let {book_name,author,} = req.body;
if(!book_name) return res.status(400).json('Book Name cant be blank');
if(!author) return res.status(400).json('Author cant be blank');
var data={book_name:book_name,
author:author};
var query = connection.query("INSERT INTO books set ? ",data,
function(err, rows)
{
if (err){
//If error
res.status(400).json('Sorry!!Unable To Add'));
console.log("Error inserting : %s ",err );
}
else
//If success
res.status(200).json('Book Added Successfully!!')
});
});
app.listen(3000, ()=> {
console.log(`app is running on port 3000`);
});
Now Let's see the Front End code on React Js:
import React from 'react';
export default class AddBook extends React.Component {
constructor(){
super();
this.state = {
bookname:'',
author:'',
};
}
updateInfo = (event) =>{
let fieldName = event.target.name;
let fieldValue = event.target.value;
if(fieldName === 'bookname') {
this.setState({bookname: fieldValue});
}
else if(fieldName === 'author'){
this.setState({author:fieldValue});
}
};
addBook=(e)=>{
let {bookname,author}=this.state;
fetch('localhost:3000/add_book', {
method: 'post',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify({
bookname:bookname,
author:author,
})
}).then(response=>response.json()).then(data=>{
window.alert(data)
//Do anything else like Toast etc.
})
}
render(){
return(
<div className="add_book">
<div>
<label>Book Name</label>
<input onChange={this.updateInfo} name="bookname" value{this.state.bookname}/>
</div>
<div>
<label >Author</label>
<input onChange={this.updateInfo} name="author" value={this.state.author}/>
</div>
<button onClick={this.addBook}>Add</button>
</div>
)
}
}
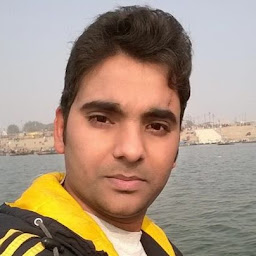
Author by
Himanshu Pandey
Updated on September 27, 2020Comments
-
Himanshu Pandey over 3 years
I want to get the data from front end react js form and insert in to mysql database using backend express. Can you tell me the flow from front end to backend with simple one field form using react js and then insert into database.