Reading JsValue in Scala
10,124
Solution 1
Quick and dirty (with no validation):
((jsonObject \ "residents").as[Seq[JsObject]].head \ "name").as[String]
Solution 2
You can do this a few ways.
Examples of class mapping and direct json field access
import play.api.libs.json.{JsError, JsSuccess, Json}
case class JsonSchemaView(name: String, location: Location, residents: Seq[Resident])
case class Location(lat: Double, long: Double)
case class Resident(name: String, age: Int, role: Option[String])
object Location {
implicit val locationFormat = Json.format[Location]
}
object Resident {
implicit val residentFormat = Json.format[Resident]
}
object JsonSchemaView {
implicit val jsonSchemaViewFormat = Json.format[JsonSchemaView]
}
object Runner {
def main (args: Array[String]) {
val mySchemaView = JsonSchemaView("name", Location(40, -40), Seq(Resident("ress", 4, None), Resident("josh", 16, Option("teenager"))))
val json = Json.toJson(mySchemaView)
println(Json.prettyPrint(json))
val myParsedSchema = Json.parse(json.toString).validate[JsonSchemaView]
myParsedSchema match {
case JsSuccess(schemaView, _) =>
println(s"success: $schemaView")
case error: JsError =>
println(error)
}
//The hard way
val jsonObject = Json.parse(json.toString())
(jsonObject \ "name").validate[String] match {
case JsSuccess(name, _) =>
println(s"success: $name")
case error: JsError =>
println(error)
}
(jsonObject \ "residents").validate[Seq[JsObject]] match {
case JsSuccess(residents, _) =>
residents foreach { resident =>
println((resident \ "age").as[Int]) //unsafe, using the wrong type will cause an exception
}
case error: JsError =>
println(error)
}
}
}
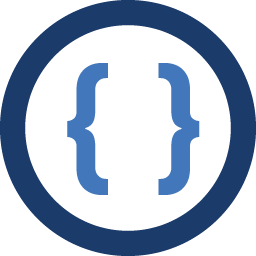
Author by
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
How do I access the name of the first resident? Here is the Json file. What comes after the following?
val bigwig = (json \ "residents")(1)..... import play.api.libs.json._ val json: JsValue = Json.parse(""" { "name" : "Watership Down", "location" : { "lat" : 51.235685, "long" : -1.309197 }, "residents" : [ { "name" : "Fiver", "age" : 4, "role" : null }, { "name" : "Bigwig", "age" : 6, "role" : "Owsla" } ] } """)