Removing punctuation in lists in Python
Solution 1
import string
punct = set(string.punctuation)
''.join(x for x in 'a man, a plan, a canal' if x not in punct)
Out[7]: 'a man a plan a canal'
Explanation: string.punctuation
is pre-defined as:
'!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'
The rest is a straightforward comprehension. A set
is used to speed up the filtering step.
Solution 2
I found a easy way to do it:
punctuation = ['(', ')', '?', ':', ';', ',', '.', '!', '/', '"', "'"]
str = raw_input("Type in a line of text: ")
for i in punctuation:
str = str.replace(i,"")
print str
With this way you will not get any error.
Solution 3
punctuation=['(', ')', '?', ':', ';', ',', '.', '!', '/', '"', "'"]
result = ""
for character in str:
if(character not in punctuation):
result += character
print result
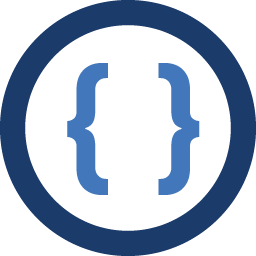
Admin
Updated on July 18, 2022Comments
-
Admin almost 2 years
Creating a Python program that converts the string to a list, uses a loop to remove any punctuation and then converts the list back into a string and prints the sentence without punctuation.
punctuation=['(', ')', '?', ':', ';', ',', '.', '!', '/', '"', "'"] str=input("Type in a line of text: ") alist=[] alist.extend(str) print(alist) #Use loop to remove any punctuation (that appears on the punctuation list) from the list print(''.join(alist))
This is what I have so far. I tried using something like:
alist.remove(punctuation)
but I get an error saying something likelist.remove(x): x not in list
. I didn't read the question properly at first and realized that I needed to do this by using a loop so I added that in as a comment and now I'm stuck. I was, however, successful in converting it from a list back into a string.