Return character from ASCII code in Windows Batch
10,397
Solution 1
@echo off
setlocal enableDelayedExpansion
set char=A
for /L %%a in (32,1,126) do (
cmd /c exit %%a
echo !=exitcodeAscii!
if "!=exitcodeAscii!" EQU "%char%" echo %%a
)
Two little changes:
a) using delayed expansion (you already enabled it)
b) qoutes around the if
arguments to prevent syntax errror with empty varaibles (especially here the space for %%a = 32
, which if
can't handle on it's own).
Solution 2
Here is the boss. Just read, fart, and applause.
cls
@echo off
cd /d "%~dp0"
chcp 65001 >nul
set title_script=char to hex
title %title_script%
rem Encode this file script in UTF-8 with BOM and let the first line blank
set ansi_code_page=1252
rem 1250 Central European
rem 1251 Cyrillic
rem 1252 Western European (Latin 1)
rem 1253 Greek
rem 1254 Turkish
rem 1255 Hebrew
rem 1256 Arabic
rem 1257 Baltic
rem 1258 Vietnamese
chcp %ansi_code_page% >nul
set /p character=Character =
set "character_ascii="
call :function_char_to_ascii character character_ascii
echo Character %character% = %character_ascii%
pause
exit
:function_char_to_hex
setlocal enabledelayedexpansion
set character=!%~1!
set tmp_file=%~dpn0_tmp.txt
echo !character!> "%tmp_file%"
set tmp_0_file=%~dpn0_tmp_0.txt
for %%a in ("%tmp_file%") do fsutil file createnew "%tmp_0_file%" %%~za >nul
set "character_hex="
for /f "skip=1 tokens=2" %%a in ('fc /B "%tmp_file%" "%tmp_0_file%"') do set "character_hex=!character_hex!%%a"
del /f /q "%tmp_file%"
del /f /q "%tmp_0_file%"
if /i "%character_hex:~-4%" == "0D0A" set character_hex=%character_hex:~0,-4%
endlocal & set %~2=%character_hex%
goto :eof
:function_change_base_number
setlocal enabledelayedexpansion
set /a input_base=%~1
set input_number=!%~2!
set /a output_base=%~3
for %%i in ("a=A" "b=B" "c=C" "d=D" "e=E" "f=F" "g=G" "h=H" "i=I" "j=J" "k=K" "l=L" "m=M" "n=N" "o=O" "p=P" "q=Q" "r=R" "s=S" "t=T" "u=U" "v=V" "w=W" "x=X" "y=Y" "z=Z") do (
call set "input_number=!input_number:%%~i!"
)
set map=0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ
set map_base=!map:~0,%input_base%!
set /a position=0
set input_number_check=%input_number%
:loop_1
set current_map_base_character=!map_base:~%position%,1!
set input_number_check=!input_number_check:%current_map_base_character%=!
if "!input_number_check!" == "" goto next_1
set /a position+=1
if "!map_base:~%position%,1!" NEQ "" goto loop_1
echo Error. Invalid character in base %input_base%.
echo.
pause
exit
:next_1
set /a carry_flag=1
set "input_number_tmp=%input_number%"
set "result_decimal="
:loop_2
set "input_number_tmp_digit=%input_number_tmp:~-1%"
if %input_number_tmp_digit% LEQ 9 goto next_2
for /l %%i in (10,1,%input_base%) do (
if /i "%input_number_tmp_digit%" EQU "!map:~%%i,1!" (
set "input_number_tmp_digit=%%i"
goto :next_2
)
)
echo Error.
:next_2
set /a result_decimal+=input_number_tmp_digit * carry_flag
set /a carry_flag *= input_base
set "input_number_tmp=%input_number_tmp:~0,-1%"
if defined input_number_tmp goto :loop_2
set /a input_number_tmp=%result_decimal%
set "result="
:loop_3
set /a "division_remainder=input_number_tmp %% !output_base!"
set /a "input_number_tmp /= !output_base!"
set result=!map:~%division_remainder%,1!%result%
if "%input_number_tmp%" NEQ "0" goto loop_3
endlocal & set %~4=%result%
goto :eof
:function_char_to_ascii
setlocal enabledelayedexpansion
set "character=!%~1!"
set "character_hex="
set "character_dec="
rem Character " is forbidden
if "%character%" == " " (
set "character_hex=20"
set "character_dec=32"
)
rem On this line, write a tabulation between the quotes, not 4 spaces.
if "%character%" == " " (
set "character_hex=9"
set "character_dec=9"
)
if "%character%" == ")" (
set "character_hex=29"
set "character_dec=41"
)
if "%character_hex%" == "" (
set "character_hex="
call :function_char_to_hex character character_hex
set "character_dec="
call :function_change_base_number 16 character_hex 10 character_dec
)
rem echo %character% = %character_dec% (x%character_hex%)
endlocal & set %~2=%character_dec%
goto :eof
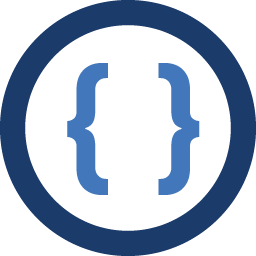
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I made a program for coding text strings in Batch. Now, I have to convert the ASCII code to a character. In reality, I have to do the opposite. I divided the chars of the strings and I want to translate it to ASCII. I found a good command to convert ASCII to char:
cmd /c exit 65 echo %=exitcodeAscii%
But, when I run it in a for cycle it stops working. With the for iteration I verify if the ASCII code (the for index) is the same of the character.
@echo off setlocal enableDelayedExpansion set char=A for /L %%a in (32,1,126) do ( cmd /c exit %%a echo %=exitcodeAscii% if %=exitcodeAscii% EQU %char% echo %%a )
It seems that the cmd doesn't work. How can I resolve this?