Run Laravel 5 seeder programmatically instead of from CLI
13,229
Solution 1
You can use the following method:
Artisan::call('db:seed');
To get the output of the last run command, you can use:
Artisan::output();
Solution 2
You can also call directly the Seeder class if needed. Just make sure you did a composer dump-autoload if you created your seeder manually.
From there code is very straightforward :
$seeder = new YourSeederClass();
$seeder->run();
Solution 3
You can add parameters to the seeder on run
example
$newSchool = School::create($data);
$schoolMeals = new \MealSeeder();
$schoolMeals->run($newSchool->id);
//school meal
public function run($school = 1)
{
$time = Carbon::now();
App\Meal::create([
'school_id' => $school,
'name' => 'Breakfast',
'slug' => 'breakfast',
'description' => 'Test Meal',
'start_time' => $time->toTimeString(),
'end_time' => $time->addMinutes(60)->toTimeString(),
]);
App\Meal::create([
'school_id' => $school,
'name' => 'Lunch',
'slug' => 'lunch',
'description' => 'Test Meal',
'start_time' => $time->toTimeString(),
'end_time' => $time->addMinutes(60)->toTimeString(),
]);
App\Meal::create([
'school_id' => $school,
'name' => 'Supper',
'slug' => 'supper',
'description' => 'Test Meal',
'start_time' => $time->toTimeString(),
'end_time' => $time->addMinutes(60)->toTimeString(),
]);
}
Related videos on Youtube
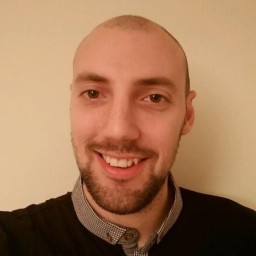
Author by
geoffs3310
Computer Science BSc 2.1 Degree. Full Time web developer 3 years experience. OOPHP5, MySql, CakePHP, Codeigniter, MVC, javascript, jquery, wordpress, opencart, interspire, HTML, CSS
Updated on September 14, 2022Comments
-
geoffs3310 3 months
Is there a way to run the Laravel 5 seeder from within PHP rather than from the command line. The hosting I am using doesn't allow me to use the command line. Just to confirm I want to do the equivalent of this but in my app code:
php artisan db:seed
-
Kirkland over 7 yearsRelated: How do you call this for a specific class? For example, if you want to call the equivalent of
php artisan db:seed --class=User
? I see that you pass an array to theArtisan::call()
function, but I get an invalid argument exception when I useArtisan::call('db:seed', ['class'=>'Users'])
saying "The 'class' argument does not exist." -
Cameron over 6 yearsArtisan::call('command:name', array('argument' => 'foo', '--option' => 'bar')); See laravel.com/docs/4.2/commands#calling-other-commands
-
Thomas about 5 yearsThe answer below is a much better option
-
alvaropgl about 3 yearsCaution if using Seeder::command() or Seeder:container(). Its its not initilized on constructor call. I have used instead from my seeder to call another seeder the method Seeder::resolve($className) as it setups $container and $command instances
-
Sven almost 3 years@Kirkland, If you want to run specific seeder Class, try
Artisan::call('db:seed', ['--class' => 'YourSeederClass']);
-
PhillipMcCubbin over 1 yearTo do it in 1 line: (new MySeeder)->run();