Sending EMail from my Javascript App via GMail API - mail appears in GMail sent list, but isn't delivered to destination email address
Your code is doing an insert(). Do a send() instead:
var path = "gmail/v1/users/me/messages/send?key=" + CLIENT_ID;
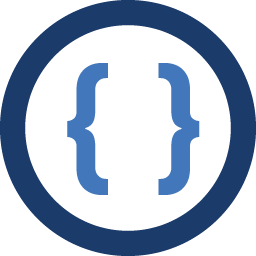
Admin
Updated on June 26, 2022Comments
-
Admin about 2 years
I've been writing a client (Chrome browser) App that integrates with GMail via the REST API. My app is written in Javascript/Angular and most of the GMail integration works fine. It can fetch from GMail - emails, profiles, labels, etc.
I'm not able to send emails I create. However, the emails I try to send do appear in the GMail sent list and, if I modify the email by adding the 'INBOX' label, they also appear in the GMail inbox. But none of the emails make it to their destination. I've been testing with several email accounts - Hotmail, Yahoo and another GMail account. Emails are never delivered to their destinations - I've checked the inboxes, spam, etc.
My code is below ... Function 'initializeGMailInterface' is run first (via the User Interface) to authorize and then the 'sendEmail' function (also via the User Interface). The code seems to track with examples I've seen and the documentation Google provides for their REST API. Authentication seems to work OK - and as I mentioned, I'm able to fetch emails, etc.
How do I get the emails to their destination?
var CLIENT_ID = '853643010367revnu8a5t7klsvsc5us50bgml5s99s4d.apps.googleusercontent.com'; var SCOPES = ['https://mail.google.com/', 'https://www.googleapis.com/auth/gmail.send', 'https://www.googleapis.com/auth/gmail.modify', 'https://www.googleapis.com/auth/gmail.labels']; function handleAuthResult(authResult) { if (authResult && !authResult.error) { loadGmailApi(); } } $scope.initializeGMailInterface = function() { gapi.auth.authorize({ client_id: CLIENT_ID, scope: SCOPES, immediate: true }, handleAuthResult); }; function loadGmailApi() { gapi.client.load('gmail', 'v1', function() { console.log("Loaded GMail API"); }); } $scope.sendEmail = function() { var content = 'HELLO'; // I have an email account on GMail. Lets call it '[email protected]' var sender = '[email protected]'; // And an email account on Hotmail. Lets call it '[email protected]'\ // Note: I tried several 'receiver' email accounts, including one on GMail. None received the email. var receiver = '[email protected]'; var to = 'To: ' +receiver; var from = 'From: ' +sender; var subject = 'Subject: ' +'HELLO TEST'; var contentType = 'Content-Type: text/plain; charset=utf-8'; var mime = 'MIME-Version: 1.0'; var message = ""; message += to +"\r\n"; message += from +"\r\n"; message += subject +"\r\n"; message += contentType +"\r\n"; message += mime +"\r\n"; message += "\r\n" + content; sendMessage(message, receiver, sender); }; function sendMessage(message, receiver, sender) { var headers = getClientRequestHeaders(); var path = "gmail/v1/users/me/messages?key=" + CLIENT_ID; var base64EncodedEmail = btoa(message).replace(/\+/g, '-').replace(/\//g, '_'); gapi.client.request({ path: path, method: "POST", headers: headers, body: { 'raw': base64EncodedEmail } }).then(function (response) { }); } var t = null; function getClientRequestHeaders() { if(!t) t = gapi.auth.getToken(); gapi.auth.setToken({token: t['access_token']}); var a = "Bearer " + t["access_token"]; return { "Authorization": a, "X-JavaScript-User-Agent": "Google APIs Explorer" }; }