Set the width of children to fill the parent
Solution 1
You can achieve this using flexbox properties.
Here is a demo:
.parent {
display: flex;
height: 120px;
background: #000;
padding: 10px;
box-sizing: border-box;
}
.child {
height: 100px;
background: #ddd;
flex: 1;
margin: 0 10px;
}
<div class="parent">
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
</div>
Solution 2
You can make the parent a flexbox
and define for the children to grow when there is space available. I removed the width for .child
.
.parent {
width: 100%;
display: inline-flex;
height: 120px;
background: #000;
padding: 10px;
box-sizing: border-box;
}
.child {
display: inline-block;
margin-left: 1%;
height: 100px;
background: #ddd;
flex-grow: 1;
}
<div class="parent">
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
</div>
Solution 3
here is the code below , i think this may help for you
.parent {
display: -webkit-flex; /* Safari */
display: flex;
height: 120px;
background: #000;
padding: 10px;
box-sizing: border-box;
}
.child {
-webkit-flex: 1; /* Safari 6.1+ */
-ms-flex: 1; /* IE 10 */
flex: 1;
margin-left: 1%;
background: #ddd;
}
<div class="parent">
<div class="child"></div>
</div><br>
<div class="parent">
<div class="child"></div>
<div class="child"></div>
</div><br>
<div class="parent">
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
</div>
Solution 4
Use flexbox:
.parent {
width: 100%;
display: flex;
background: #000;
padding: 10px;
box-sizing: border-box;
margin-bottom: 15px;
}
.child {
flex: 1;
margin: 0 10px;
height: 100px;
background: #ddd;
}
<div class="parent">
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
<div class="child"></div>
</div>
<div class="parent">
<div class="child"></div>
</div>
Solution 5
You are using width like 30% which is fixed for every element, so every time you create other element its size is fixed and added at the end of residing elements and after total width is more than that of parent container it overflows.
Instead use flex-box.
.parent {
width: 100%;
display:flex;
height: 120px;
background: #000;
padding: 10px;
box-sizing: border-box;
}
.child {
flex:1;
margin-left: 1%;
width: 31.4%;
height: 100px;
background: #ddd;
}
<div class="parent">
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
<div class="child"></div>
</div>
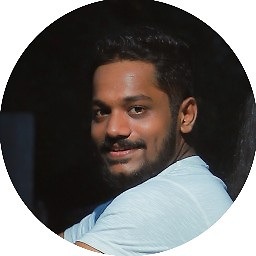
Jithin Raj P R
I am a learner, still learning and will try to learn till my last key stroke..!! Happy to help and appreciate if shown right way...!! Thats all about me, so don't wait lets start learning something new together...!!
Updated on June 12, 2022Comments
-
Jithin Raj P R about 1 year
I want the children of the
div
fill its width.now am using a code like this:
.parent { width: 100%; display: inline-block; height: 120px; background: #000; padding: 10px; box-sizing: border-box; } .child { display: inline-block; margin-left: 1%; width: 31.4%; height: 100px; background: #ddd; }
<div class="parent"> <div class="child"></div> <div class="child"></div> <div class="child"></div> </div>
and it's working for
3 boxes
, but what I want is that - Even if the box count isone
ortwo
i want them to fill the parent width. I want to achieve this using only CSS.