Swift 4.2 Setter Getter, All paths through this function will call itself
Solution 1
Your issue is that there is no stored property type
for the getter to return. type
is a computed property. When you try to read its value, it calls the getter you defined. This getter calls the getter, which in turn calls the getter which calls the getter... and so on. You have infinite recursion.
Most likely, what you meant to do is have a stored property, that just has some fancy behaviour whenever its set. Rather than using a computed property with a custom get
and set
, use a stored property with a willSet
or didSet
block:
@objc var type: DecisionType {
didSet {
let isDecisionDouble = newValue == DecisionType.DecisionDouble
okButton.isHidden = isDecisionDouble;
yesButton.isHidden = !isDecisionDouble;
noButton.isHidden = !isDecisionDouble;
}
}
Solution 2
A better approach for this case if to have one extra property which you will use it in return value for getter and you set it when your main property change.
For instance let say your main property which you use is type
then have an extra property _type
note the underscore next to it.
Then here is how you would set and retrieve your and set your main property type
// This is a an extra property which you will use internally
private var _type: DecisionType?
// Then use it as shown below
var type:DecisionType? {
get {
return _type
}
set {
_type = newValue
}
}
Related videos on Youtube
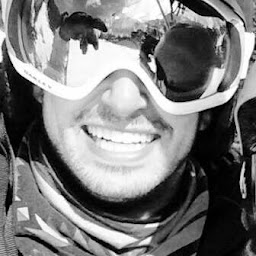
Travis Delly
Updated on September 13, 2020Comments
-
Travis Delly almost 4 years
With swift 4.2 I have begun to see a lot of issues, and one of them i'm not really sure how to resolve, since my getter method should be returning the value itself.
I imagine what is happening is that the getter will attempt to access the getter when calling self.type
How can i resolve this issue?
Here is a screenshot of the code with the error.
Thanks in advance
Here is the written code
@objc var type: DecisionType { set { if(newValue == DecisionType.DecisionDouble){ //Yes button and NO button should be showing okButton.isHidden = true; yesButton.isHidden = false; noButton.isHidden = false; } else { //Only Ok button should be showing okButton.isHidden = false; yesButton.isHidden = true; noButton.isHidden = true; } } get { return self.type; } };
-
Max0u over 5 yearsDid you manage to fix the return of self.type ?
-
Alexander over 5 years@TravisDelly Has your question been answered?
-
Travis Delly over 5 yearsye i used didSet rather than a getter and setter.
-
Alexander over 5 years@TravisDelly Then please mark the answer as accepted
-
Travis Delly over 5 years@Alexander gotchu, sorry took me so long! Thank you for the answer!
-