tensorflow.python.framework.errors_impl.NotFoundError while creating a custom inception
The problem comes from this line:
label_lines = [line.rstrip() for line
in tf.gfile.GFile("/tf_files/retrained_labels.txt")]
Please check:
- The existence of the folder
tf_files
in the root of the file system -- you can runls /tf_files
to check this; - (if item 1 is ok) if you have reading/writing permission on
/tf_files/retrained_labels.txt
.
Related videos on Youtube
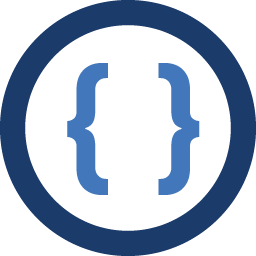
Admin
Updated on September 16, 2022Comments
-
Admin over 1 year
Im using the following code to create a custom inception using tensorflow.
import tensorflow as tf import sys interesting_class = sys.argv[1:] print("Interesting class: ", interesting_class) # Read in the image_data from os import listdir from shutil import copyfile from os.path import isfile, join varPath = 'toScan/' destDir = "scanned/" imgFiles = [f for f in listdir(varPath) if isfile(join(varPath, f))] # Loads label file, strips off carriage return label_lines = [line.rstrip() for line in tf.gfile.GFile("/tf_files/retrained_labels.txt")] # Unpersists graph from file with tf.gfile.FastGFile("/tf_files/retrained_graph.pb", 'rb') as f: graph_def = tf.GraphDef() graph_def.ParseFromString(f.read()) _ = tf.import_graph_def(graph_def, name='') with tf.Session() as sess: # Feed the image_data as input to the graph and get first prediction softmax_tensor = sess.graph.get_tensor_by_name('final_result:0') file_count = len(imgFiles) i = 0 for imageFile in imgFiles: print("File ", i, " of ", file_count) i = i+1 image_data = tf.gfile.FastGFile(varPath+"/"+imageFile, 'rb').read() print (varPath+"/"+imageFile) predictions = sess.run(softmax_tensor, \ {'DecodeJpeg/contents:0': image_data}) # Sort to show labels of first prediction in order of confidence top_k = predictions[0].argsort()[-len(predictions[0]):][::-1] firstElt = top_k[0]; newFileName = label_lines[firstElt] +"--"+ str(predictions[0][firstElt])[2:7]+".jpg" print(interesting_class, label_lines[firstElt]) if interesting_class == label_lines[firstElt]: print(newFileName) copyfile(varPath+"/"+imageFile, destDir+"/"+newFileName) for node_id in top_k: human_string = label_lines[node_id] score = predictions[0][node_id] print (node_id) print('%s (score = %.5f)' % (human_string, score))
Im getting the following error while executing this
('Interesting class: ', []) Traceback (most recent call last): File "/Users/Downloads/imagenet_train-master/label_dir.py", line 22, in in tf.gfile.GFile("/tf_files/retrained_labels.txt")] File "/Users/tensorflow/lib/python2.7/site-packages/tensorflow/python/lib/io/file_io.py", line 156, in next retval = self.readline() File "/Users/tensorflow/lib/python2.7/site-packages/tensorflow/python/lib/io/file_io.py", line 123, in readline self._preread_check() File "/Users/tensorflow/lib/python2.7/site-packages/tensorflow/python/lib/io/file_io.py", line 73, in _preread_check compat.as_bytes(self.name), 1024 * 512, status) File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/contextlib.py", line 24, in __exit self.gen.next() File "/Users/tensorflow/lib/python2.7/site-packages/tensorflow/python/framework/errors_impl.py", line 466, in raise_exception_on_not_ok_status pywrap_tensorflow.TF_GetCode(status)) tensorflow.python.framework.errors_impl.NotFoundError: /tf_files/retrained_labels.txt
Why am I getting this error?
Following is my folder structure:
tensorflow_try |- new_pics | |- class1 | |- class2 | |- ... |- toScan |- scanned
-
cyril almost 7 yearsI had this same issue and had to make a small update to the label_image.py file. The line that Micael mentioned was missing a
/
in front oftf_files
on the Tensorflow for Poets site.