Uniformly distributed data in d dimensions
Solution 1
Assuming independence of the individual coordinates, then the following will generate a random point in [-1, 1)^d
np.random.random(d) * 2 - 1
The following will generate n
observations, where each row is an observation
np.random.random((n, d)) * 2 - 1
Solution 2
As has been pointed out, randn produces normally distributed number (aka Gaussian). To get uniformly distributed you should use "uniform".
If you just want a single sample at a time of 10 uniformly distributed numbers you can use:
import numpy as np
x = np.random.uniform(low=-1,high=1,size=10)
OR if you'd like to generate lots (e.g. 100) of them at once then you can do:
import numpy as np
X = np.random.uniform(low=-1,high=1,size=(100,10))
Now X[0], X[1], ... each has length 10.
Solution 3
Without numpy:
[random.choice([-1,1]) for _ in range(N)]
There may be reasons to use numpy's internal mechanisms, or use random()
manually, etc. But those are implementation details, and also related to how the random number generation rations the bits of entropy the operating system provides.
Solution 4
You can import the random
module and call random.random
to get a random sample from [0, 1). You can double that and subtract 1 to get a sample from [-1, 1).
Draw d values this way and the tuple will be a uniform draw from the cube [-1, 1)^d.
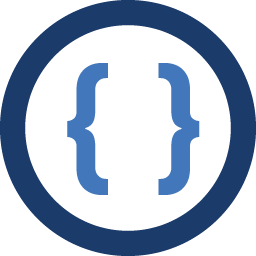
Admin
Updated on June 08, 2022Comments
-
Admin almost 2 years
How can I generate a uniformly distributed [-1,1]^d data in Python? E.g. d is a dimension like 10.
I know how to generate uniformly distributed data like np.random.randn(N) but dimension thing is confused me a lot.
-
ninjagecko about 12 yearsHmm, I think I was downvoted by someone because the OP did not clearly state he was looking for a uniform distribution of the form [-1,1]x[-1,1]x...x[-1,1], not a uniform distribution amongst the values of [-1,-1]^d (that is, {-1,1}^d). Since I feel the OP's question was extremely vague (OP thinks that the function they want is uniform when it is actually a gaussian), and that the syntax was not clear, and that my interpretation is reasonable, I'll just leave this here in case someone else finds it useful.
-
Abinash Dash over 3 yearsThanks. got exactly what I expected. I needed 2d samples in range -5 to 5 . So, I used np.random.random((n,2)) * 10 - 5.