ValueError: invalid literal for int() with base 10 -- how to guard against invalid user input?
Solution 1
Well, there really a way to 'fix' this, it is behaving as expected -- you can't case a letter to an int, that doesn't really make sense. Your best bet (and this is a pythonic way of doing things), is to simply write a function with a try... except block:
def get_user_number():
i = input("Enter a number.\n")
try:
# This will return the equivalent of calling 'int' directly, but it
# will also allow for floats.
return int(float(i))
except ValueError:
#Tell the user that something went wrong
print("I didn't recognize {0} as a number".format(i))
#recursion until you get a real number
return get_user_number()
You would then replace these lines:
z = input("Enter a number.\n")
z = int(z)
with
z = get_user_number()
Solution 2
Try checking
if string.isdigit(z):
And then executing the rest of the code if it is a digit.
Because you count up in intervals of one, staying with int() should be good, as you don't need a decimal.
EDIT: If you'd like to catch an exception instead as wooble suggests below, here's the code for that:
try:
int(z)
do something
except ValueError:
do something else
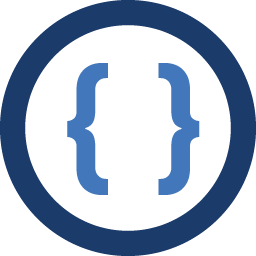
Admin
Updated on October 24, 2021Comments
-
Admin over 2 years
I made a program where the user enters a number, and the program would count up to that number and display how much time it took. However, whenever I enter letters or decimals (i.e. 0.5), I would get a error. Here is the full error message:
Traceback (most recent call last): File "C:\Documents and Settings\Username\Desktop\test6.py", line 5, in <module> z = int(z) ValueError: invalid literal for int() with base 10: 'df'
What can I do to fix this?
Here is the full code:
import time x = 0 print("This program will count up to a number you choose.") z = input("Enter a number.\n") z = int(z) start_time = time.time() while x < z: x = x + 1 print(x) end_time = time.time() diff = end_time - start_time print("That took",(diff),"seconds.")
Please help!