Why are my jQuery animations choppy in webkit-based browsers?
Solution 1
For me, it didn't make a difference if it was 24-bit PNGs, 8-bit PNGs, GIFs, JPEGs, etc., if there was a large image on the screen there were issues with my animations. If you have elements z-index'ed above a large image, try adding this CSS to your element:
-webkit-transform: translateZ(0);
For me, it worked, but it left animation artifacts.
What finally solved it for me was to simply change this:
$('#myimage').animate({
height: 0,
top: '-=50'
}, 500, 'linear');
To this:
$('#myimage').animate({
height: '-=1'
}, 1, 'linear').animate({
height: 0,
top: '-=50'
}, 500, 'linear');
I just added a small 1 millisecond animation onto the beginning. My thinking was it would possibly "prep" Chrome for the real animation coming up, and it worked. You may want to change it to 20 or 50 milliseconds to be safe.
Solution 2
In my case, the issue wasn't with the code, but with the images--specifically, large images that have been (forcibly) scaled down via the css width property. Of course larger images might take more processing to animate, but IE and FF seems to handle them just fine scaled down (in my case, images 2000px wide were scaled to be 800px wide). Meanwhile, it appears that Chrome and Safari get bogged down animating such images. Once I batch-shrunk all my images in Photoshop to actually be 800px wide, the animations were smooth everywhere.
Other details. I'm using jQuery 1.7. I encountered the issue for animating the img element itself, as well as a div element with a background image.
Solution 3
I realize this thread is old, but in the interest of efficient coding..., I ran into a similar issue with choppy animations using Chrome recently and in searching for a solution, came across this thread(but not FF or IE) traced the issue to one of my collapse icons using a png as opposed to a gif (as soon as I swapped the png for a gif) the webkit browser had no issues producing smooth animations (but as soon as I swapped the png back in, my animations were once again choppy...)
Related videos on Youtube
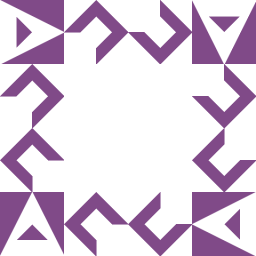
Andrew Cheong
Early web dev (1999 to around when jQuery came out), a decade of C++ in stock exchanges, and now Java in ad tech. Here, I used to go by A—— C——, farmed XP on the regex tag to wield fake authority on unrelated subjects, ♦'ed a beta site, and created the SOCVR chatroom. (I take no credit for the community it's grown into today, that was @rene et al.)
Updated on June 04, 2022Comments
-
Andrew Cheong 6 months
I'm working with a very simple animation: sliding a line of images to the left:
$('#button').click( function() { $('div#gallery').animate({scrollLeft:'+=800px'}, 1000); } );
(It's supposed to be an image gallery; I hide the overflow to show only one image at a time.)
Anyway, despite trying various easing parameters, even at slow speeds the animation was very choppy in Chrome and Safari, but always smooth in Internet Explorer and Firefox. (Most issues raised online are about IE or Firefox being choppy!)
I found the cause, for me. It's a very special case that probably won't apply to most, but maybe it'll help someone regardless. I'll post my answer below. (The site guidelines allow this, I think.)
-
Sparky almost 11 yearsYes, ideally if/when you solve your own question, you post it below and "accept" your own answer... as long as it's on-topic for this site and presented clear enough to help future readers. My only suggestion would be that you post an example & code within your question above to give it more context.
-
-
Sparky over 10 yearsDid your png and gif have transparency?
-
JAMES over 10 yearsIt did not as it was it an 8 x 8 box (portrayed a collapse and expand icon as a rectangle and square)
-
Andrew Cheong over 10 yearsOld or new, issues like these can always use more solutions. Thanks for chiming in. I was using jpg's at the time, personally.
-
codeGEN almost 9 yearsthanks Garvin. -webkit-transform: translateZ(0); solved my issue as well. thanks for sharing really helpful.
-
DavidT about 8 years+1, 9 months on
-webkit-transform: translateZ(0);
still helped me. Thanks. -
Sam Gurdus almost 5 yearsSuper old thread, I know, but I am having the same issue on Chrome. Problem is, my images are dynamically scaled depending on the window size. Any suggestions?