Angular : Can't export excel using ExcelJS - error TS2307: Cannot find module 'stream' - error TS2503: Cannot find namespace 'NodeJS'
Solution 1
You can't write directly a file on client side. that method is mean to be used on backend side in nodejs. if your expectation is download this file on client side. your code should be like :-
import { Component } from "@angular/core";
import * as Excel from "exceljs";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
title = "resttest10";
async downloadExcel() {
const date = new Date()
.toISOString()
.slice(0, 10)
.split("-")
.reverse()
.join("/");
console.log(date);
const workbook = new Excel.Workbook();
const worksheet = workbook.addWorksheet("My Sheet");
worksheet.columns = [
{ header: "Id", key: "id", width: 10 },
{ header: "Name", key: "name", width: 32 },
{ header: "D.O.B.", key: "dob", width: 15 }
];
worksheet.addRow({ id: 1, name: "John Doe", dob: new Date(1970, 1, 1) });
worksheet.addRow({ id: 2, name: "Jane Doe", dob: new Date(1965, 1, 7) });
workbook.xlsx.writeBuffer().then((data: any) => {
console.log("buffer");
const blob = new Blob([data], {
type:
"application/vnd.openxmlformats-officedocument.spreadsheetml.sheet"
});
let url = window.URL.createObjectURL(blob);
let a = document.createElement("a");
document.body.appendChild(a);
a.setAttribute("style", "display: none");
a.href = url;
a.download = "export.xlsx";
a.click();
window.URL.revokeObjectURL(url);
a.remove();
});
}
}
Solution 2
In your tsconfig.app.json file add "types": ["node"]
Note, that the "types" are in the compilerOptions section of the tsconfig.
Solution 3
I found a simple way to resolve this!
comment or remove the import import * as Excel from 'exceljs'
Now you can import like this:
import * as Excel from 'exceljs/dist/exceljs.min.js'
Related videos on Youtube
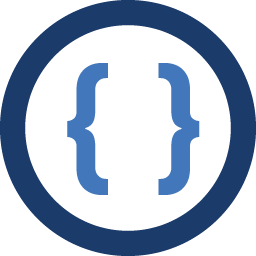
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I was try to export an excel file using ExcelJS
Here is my console in VS Code terminal :
ERROR in node_modules/exceljs/index.d.ts:1398:22 - error TS2307: Cannot find module 'stream'. 1398 read(stream: import('stream').Stream): Promise<Workbook>; ~~~~~~~~ node_modules/exceljs/index.d.ts:1424:23 - error TS2307: Cannot find module 'stream'. 1424 write(stream: import('stream').Stream, options?: Partial<XlsxWriteOptions>): Promise<void>; ~~~~~~~~ node_modules/exceljs/index.d.ts:1511:22 - error TS2307: Cannot find module 'stream'. 1511 read(stream: import('stream').Stream, options?: Partial<CsvReadOptions>): Promise<Worksheet>; ~~~~~~~~ node_modules/exceljs/index.d.ts:1531:23 - error TS2307: Cannot find module 'stream'. 1531 write(stream: import('stream').Stream, options?: Partial<CsvWriteOptions>): Promise<void>; ~~~~~~~~ node_modules/exceljs/index.d.ts:1828:19 - error TS2307: Cannot find module 'stream'. 1828 stream: import('stream').Stream; ~~~~~~~~ node_modules/exceljs/index.d.ts:1872:34 - error TS2503: Cannot find namespace 'NodeJS'. 1872 dictionary: Buffer | NodeJS.TypedArray | DataView | ArrayBuffer; // deflate/inflate only, empty dictionary by default ~~~~~~
here is app.component.html
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Example</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css"> --> <!-- serial must : first jquery then popper then bootstrap --> <!-- <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js"></script> --> </head> <body> <div class="row"> <div class="col-md-2"> <button class="btn btn-secondary" (click)='downloadExcel()'>Download as Excel</button> </div> </div> </body> </html>
here is app.component.ts
import { Component } from '@angular/core'; import * as Excel from 'exceljs'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'resttest10'; async downloadExcel() { const date = new Date().toISOString().slice(0, 10).split('-').reverse().join('/'); console.log(date); const workbook = new Excel.Workbook(); const worksheet = workbook.addWorksheet('My Sheet'); worksheet.columns = [ { header: 'Id', key: 'id', width: 10 }, { header: 'Name', key: 'name', width: 32 }, { header: 'D.O.B.', key: 'dob', width: 15, } ]; worksheet.addRow({ id: 1, name: 'John Doe', dob: new Date(1970, 1, 1) }); worksheet.addRow({ id: 2, name: 'Jane Doe', dob: new Date(1965, 1, 7) }); // save under export.xlsx await workbook.xlsx.writeFile('export.xlsx'); // load a copy of export.xlsx const newWorkbook = new Excel.Workbook(); await newWorkbook.xlsx.readFile('export.xlsx'); const newworksheet = newWorkbook.getWorksheet('My Sheet'); newworksheet.columns = [ { header: 'Id', key: 'id', width: 10 }, { header: 'Name', key: 'name', width: 32 }, { header: 'D.O.B.', key: 'dob', width: 15, } ]; await newworksheet.addRow({ id: 3, name: 'New Guy', dob: new Date(2000, 1, 1) }); await newWorkbook.xlsx.writeFile('export2.xlsx'); console.log('File is written'); } }
I don't get it why it is looking for stream module (and more funny thing - Cannot find namespace 'NodeJS' - I can run all other angular NodeJS projects without error)
Please point out why i can't export excel.
-
Admin almost 4 yearsi did it. no change
-
Admin almost 4 years+1 for solving my previous state, now at last angular runs on the same code, but still can't save files //core.js:6228 ERROR Error: Uncaught (in promise): TypeError: a.createWriteStream is not a function TypeError: a.createWriteStream is not a function//
-
Admin almost 4 yearsI guess its better than my version, but it generates a new error TypeError: a.createWriteStream is not a function TypeError: a.createWriteStream is not a function at e.value (exceljs.min.js:3) at AppComponent.<anonymous> (app.component.ts:31) however, at line 31, await workbook.xlsx.writeFile('export.xlsx'); it seems for some reason its not executing (paste.ubuntu.com/p/dc83RdDmPJ)
-
Aakash Garg almost 4 yearsi am not using writeFile, did you closely see? I am using writeBuffer. writeFile is only applicable for backend.
-
Admin almost 4 yearsoh i get it now, Please edit your answer remove those lines i guess // save under export.xlsx await workbook.xlsx.writeFile('export.xlsx'); When I use only writeBuffer , all is fine.
-
Aakash Garg almost 4 yearsand please mark it as answer if it resolved your issue.
-
piupaul over 3 yearscould you please inform which version of excelJs you are using?
-
Admin over 3 years@piupaul 3.9.0 exceljs
-
Admin over 3 years@AakashGarg so your above solution is correct. a lof of thanks. But I want to make the above file .csv for a project (not xlsx) . i tried changing your this line to csv a.download = "export.csv"; it says file corrupted then. So How Can I convert the exact above example to csv ?
-
Olga almost 3 yearsThis seems to be a duplicate for an existing anwser.
-
Elson Costa almost 3 yearscomment or remove the import "import * as Excel from 'exceljs'" Now you can import like this: import * as Excel from 'exceljs/dist/exceljs.min.js';