Angular - Dynamically loading an image
Solution 1
Webpack uses url-loader
to load images from your angular project that comes with extension .png,.jpg
etc . Take a look at the below code from the url-loader
repo in github https://github.com/webpack-contrib/url-loader
module.exports = {
module: {
rules: [
{
test: /\.(png|jpg|gif)$/,
use: [
{
loader: 'url-loader',
options: {
limit: 8192
}
}
]
}
]
}
}
it looks for image files with the above extensions. But we need to use require
statement to the actual image path for the Webpack to understand the path of the image so that it can pick them up. This is required in .ts
/ typescript files while using the path of the image as a variable or returning the path value from a method . The first image tag
<img src="../../assets/timeblockr-logo.png" />
was working because Webpack uses html-loader
for .html
files, which automatically replaces src attribute values on image tags with the bundled image URL.
So your getLogo()
method should be like below
getLogo():string {
return require("../assets/timeblockr-logo.png");
}
Solution 2
Your image source should be without ../../
. The image paths are relative in the output folder. Just change to this:
<img src="assets/timeblockr-logo.png" />
<img [src]="customerservice.getLogo()" />
And your function should be:
getLogo(): string {
return "assets/timeblockr-logo.png";
}
As per your comments, you need to change your "assets"
entry in .angular-cli.json
to this:
"assets": [
"assets",
"favicon.ico",
{
"glob": "**/*",
"input": "./assets/images/",
"output": "./assets/images/",
"allowOutsideOutDir": false
}
],
Solution 3
Change <img [src]="customerservice.getLogo()" />
To <img [attr.src]="customerservice.getLogo()" />
Read more about Attribute Binding.
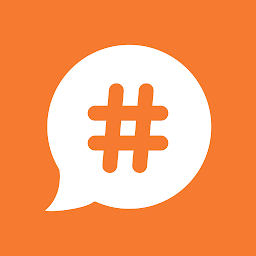
Rick - HashtagNetwork
Updated on June 13, 2022Comments
-
Rick - HashtagNetwork almost 2 years
I've searched all over StackOverflow but I haven't been able to find a solution to my problem.
F.Y.I I'm using ASP.net Core 2.0's default angular project
I'm currently building an Angular application where I'm trying to load in a customer's logo based on a config found elsewhere.
I'm trying to load this file into my home.component.html
I created a "CustomerService" which has a function getLogo() which in itself returns the customer's logo's path.
My folder structure is as follows: FOLDER STRUCTURE IMAGE
The assets folder holds the images, and the HTML found below is found in home.component.htmlI know that "../../" isn't the way to go, so my first question is how I'd be able to fix that.
I've created the following HTML structure:
<img src="../../assets/timeblockr-logo.png" /> <img [src]="customerservice.getLogo()" />
And the CustomerService's getLogo function returns the following:
getLogo() { return "../../assets/timeblockr-logo.png"; }
** EDIT: FOUND THE SOLUTION!!! **
Turns out I had to use:
getLogo(): string{ return require("../assets/timeblockr-logo.png"); }
Special thanks to @Niladri for the solution to this issue!
-
Rick - HashtagNetwork about 6 yearsThis results in localhost:56982/assets/timeblockr-logo.png 404 (Not Found) it seems like webpack doesn't update this url. because I also added the other image include (which does work) and it's SRC is changed to dist/a0eeffbba7398daebf178f3d7fab50eb.png
-
Faisal about 6 years@TomaszKula ,
<img [src]="customerservice.getLogo()" />
is also correct way to specify the source. -
Rick - HashtagNetwork about 6 yearsThis results in localhost:56982/assets/timeblockr-logo.png 404 (Not Found) it seems like webpack doesn't update this url. because I also added the other image include (which does work) and it's SRC is changed to dist/a0eeffbba7398daebf178f3d7fab50eb.png
-
Niladri about 6 years@Rick-HashtagNetwork can you post your folder structure? are you using angular-cli?
-
Rick - HashtagNetwork about 6 yearsHey @Niladri , Folder structure is already in the post ;) I'm using ASP.net Core's default angular project.
-
Niladri about 6 years@Rick-HashtagNetwork can you post your angular-cli.json file
-
Rick - HashtagNetwork about 6 years@Niladri added to the post! :)
-
Rick - HashtagNetwork about 6 years@Faisal Unfortunately, this didn't do anything.
-
Rick - HashtagNetwork about 6 years@Faisal I copy and pasted all of your changes, including the string indication etc. But I'm still getting errors: SCREENSHOT
-
Rick - HashtagNetwork about 6 years@Faisal Also in this manner, the previously working image include isn't working anymore.