Configure CORS response headers on AWS Lambda?
Solution 1
If you're using {proxy+}
endpoint, you must handle CORS HTTP requests in the Lambda function. The implementation depends on the framework you're using. For Express, the easiest solution is to simply use Express CORS middleware.
If you don't want to handle CORS
requests by Lambda
, try changing the settings of your Lambda Method
to handle CORS
on the API Gateway
level.
Here's a detailed official tutorial for CORS setup on AWS API Gateway.
It's also critical that you allow header X-Api-Key
in Access-Control-Allow-Headers
otherwise auth won't work and you'll get errors.
EDIT: In November 2015 the API Gateway team added a new feature to simplify CORS setup.
Solution 2
If you have lambda-proxy enabled, you need to set the CORS headers manually:
module.exports.hello = function(event, context, callback) {
const response = {
statusCode: 200,
headers: {
"Access-Control-Allow-Origin" : "*", // Required for CORS support to work
"Access-Control-Allow-Credentials" : true // Required for cookies, authorization headers with HTTPS
},
body: JSON.stringify({ "message": "Hello World!" })
};
callback(null, response);
};
https://serverless.com/framework/docs/providers/aws/events/apigateway#enabling-cors
Solution 3
Here is a sample, I hope this helps you:
...
return {
statusCode: 200,
headers: {
"Access-Control-Allow-Headers" : "Content-Type",
"Access-Control-Allow-Origin": "*", // Allow from anywhere
"Access-Control-Allow-Methods": "GET" // Allow only GET request
},
body: JSON.stringify(response)
}
}
For more information please check this link: https://docs.aws.amazon.com/apigateway/latest/developerguide/how-to-cors.html
Solution 4
If you're using JQuery $.ajax, it will send the X-Requested-With with the POST following the OPTIONS request, so you need to make sure when setting up your OPTIONS access-control-accept-headers on AWS API to include that header: X-Requested-With along with the others.
Related videos on Youtube
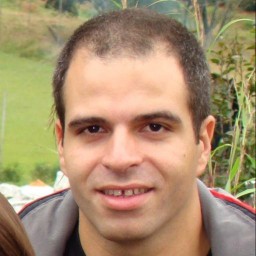
Alessandro Oliveira
AWS Certified Solutions Architect Professional, creates cost effective and scaleable solutions all kinds of workloads, have strong background in Design Patterns, JavaEE Architectures and Service Oriented Architectures.
Updated on July 09, 2022Comments
-
Alessandro Oliveira 2 months
I'm trying to create a new service using AWS API Gateway, but I found out the browser automatically calls OPTIONS method in order to obtain CORS information.
The problem is that AWS API Gateway does not offer a native way to configure CORS headers.
Is it possible to create a Lambda Script in order to respond to OPTIONS method?
-
James about 7 yearsDid you take a look at Enable CORS for a Method in API Gateway? Did that not work?
-
-
nelsonic over 6 yearswhere did you discover this? is it documented? how were you able to add the header in the API Gateway resources "Enable CORS" form?
-
Chandru almost 6 yearsenabling CORS setup in api gateway in the not enough if your api gateway is configured as lambdaproxy
-
chrismarx over 4 years@Chandru I agree, the aws docs says that for proxy setups, the response has to come from the http backend, what should this look for like for node/express?
-
adamkonrad over 4 yearsTry creating one
{proxy+}
endpoint and have all the requests forwarded to the Lambda function. -
drogon almost 4 yearsThis answer is not correct as stated above. The one below should be marked.
-
adamkonrad almost 4 yearsThis answer is correct. However Lambda-level configuration is needed for {proxy+} endpoints because all requests are just passed through from API Gateway.
-
adamkonrad over 3 years@Alisson You should see
{proxy+}
in your API Gateway resource.