Delete rows based on range of values in column
13,496
Solution 1
Or a more straight forward implementation will be just negate these rows using !
dataset[with(dataset, !((X >= 61701 & X <= 61721) | (X >= 61901 & X <= 61929))), ]
Or
dataset[with(dataset, !((X %in% 61701:61721) | (X %in% 61901:61929))), ]
For a big data set you can use data.table
s %between%
function
library(data.table)
setDT(dataset)[!(X %between% c(61701, 61721) | X %between% c(61901, 61929))]
Solution 2
You should be able to exclude those ranges by including everything less than, greater than, and in between them. Something like:
dataset[dataset$X < 61701 | dataset$X > 61929 | (dataset$X > 61721 & dataset$X < 61901),]
Or using subset
:
subset(dataset, X < 61701 | X > 61929 | (X > 61721 & X < 61901)
Solution 3
Using dplyr package:
exclude <- c(61701:61721, 61901:61929)
library(dplyr)
datasetnew <- dataset %>%
filter(!(X %in% exclude))
Related videos on Youtube
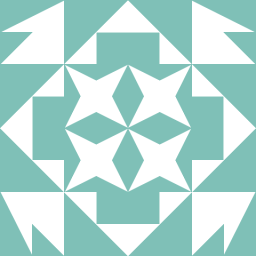
Author by
Ash
Post-doc in physical activity and public health at Durham University, UK.
Updated on October 11, 2022Comments
-
Ash less than a minute
I would like to delete rows of data in a dataframe if the values in a column (in this case a participant identification number) fall within a certain range e.g. 61701 to 61721 & 61901 to 61929.
I know how to subset data based on a threshold e.g.:
datasetnew = dataset[dataset$X<=100, ]
But not sure how to subset and remove the rows using a range of numbers. Not sure subset is what I need.
-
AshThanks David this works perfectly for subsetting the data. What I want to do is remove those particular rows from the dataframe. I should have thought it through more!
-
David ArenburgHaven't tested, but probably something simple like
dataset[(dataset$X >= 61701 & dataset$X <= 61721) | (dataset$X >= 61901 & dataset$X <= 61929),]
ordataset[dataset$X %in% 61701:61721) | (dataset$X %in% 61901:61929),]
. Probably could usewith
too in order to reducedataset
calls
-