Flutter - Character encoding is not behaving as expected
Solution 1
The problem is that your JSON is stored locally.
Let's say you have
Map<String, String> jsonObject = {"info": "Æ æ æ Ö ö ö"};
So to show your text correctly you have to encode and decode back your JSON with utf-8.
I understand that's serialization and deserialization are costly operations, but's it's a workaround for locally stored JSON objects that contains UTF-8 texts.
import 'dart:convert';
jsonDecode(jsonEncode(jsonObject))["info"]
If you get that JSON from server, then it's much more simpler, for example in dio
package you can chose contentType
params that's is "application/json; charset=utf-8" by default.
Solution 2
I had similar issues with accented characters. They were not displayed as expected. This worked for me
final codeUnits = source.codeUnits;
return Utf8Decoder().convert(codeUnits);
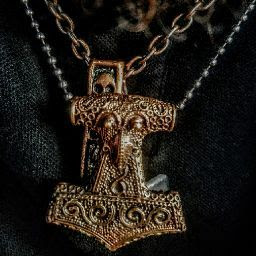
Thorvald
Updated on December 15, 2022Comments
-
Thorvald over 1 year
I am parsing a local
JSON
file that has words containing rarely used special characters in Icelandic.When displaying the characters I get mixed up symbols but not the characters, for some others I just get a square instead of a symbol.
I am using this type of encoding
"\u00c3"
Update: Example of the characters I am using: þ, æ, ý, ð
Q: What is the best way to display those kind of characters and avoid any chance of display failures?
Update #2: How I am parsing:
Future<Null> getAll() async{ var response = await DefaultAssetBundle.of(context).loadString('assets/json/dictionary.json'); var decodedData = json.decode(response); setState(() { for(Map word in decodedData){ mWordsList.add(Words.fromJson(word)); } }); }
The class:
class Words{ final int id; final String wordEn, wordIsl; Words({this.id, this.wordEn, this.wordIsl}); factory Words.fromJson(Map<String, dynamic> json){ return new Words( id: json['wordId'], wordEn: json['englishWord'], wordIsl: json['icelandicWord'] ); } }
JSON Model:
{ "wordId": 47, "englishWord": "Age", //Here's a String that has two special characters "icelandicWord": "\u00c3\u00a6vi" }