Flutter how to programmatically exit the app
Solution 1
For iOS
SystemNavigator.pop()
: Does NOT WORK
exit(0)
: Works but Apple may SUSPEND YOUR APP
Please see:
https://developer.apple.com/library/archive/qa/qa1561/_index.html
For Android
SystemNavigator.pop()
: Works and is the RECOMMENDED way of exiting the app.
exit(0)
: Also works but it's NOT RECOMMENDED as it terminates the Dart VM process immediately and user may think that the app just got crashed.
Please see:
https://api.flutter.dev/flutter/services/SystemNavigator/pop.html
Solution 2
Below worked perfectly with me in both Android
and iOS
, I used exit(0)
from dart:io
import 'dart:io';
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(widget.title),
),
body: new ... (...),
floatingActionButton: new FloatingActionButton(
onPressed: ()=> exit(0),
tooltip: 'Close app',
child: new Icon(Icons.close),
),
);
}
UPDATE Jan 2019 Preferable solution is:
SystemChannels.platform.invokeMethod('SystemNavigator.pop');
As described here
Solution 3
You can do this with SystemNavigator.pop()
.
Solution 4
Answers are provided already but please don't just copy paste those into your code base without knowing what you are doing:
If you use SystemChannels.platform.invokeMethod('SystemNavigator.pop');
note that doc is clearly mentioning:
Instructs the system navigator to remove this activity from the stack and return to the previous activity.
On iOS, calls to this method are ignored because Apple's human interface guidelines state that applications should not exit themselves.
You can use exit(0)
. And that will terminate the Dart VM process immediately with the given exit code. But remember that doc says:
This does not wait for any asynchronous operations to terminate. Using exit is therefore very likely to lose data.
Anyway the doc also did note SystemChannels.platform.invokeMethod('SystemNavigator.pop');
:
This method should be preferred over calling dart:io's exit method, as the latter may cause the underlying platform to act as if the application had crashed.
So, keep remember what you are doing.
Solution 5
you can call this by checking platform dependent condition. perform different action on click for android and ios.
import 'dart:io' show Platform;
import 'package:flutter/services.dart';
RaisedButton(
onPressed: () {
if (Platform.isAndroid) {
SystemNavigator.pop();
} else if (Platform.isIOS) {
exit(0);
}
},
child: Text("close app")
)
Related videos on Youtube
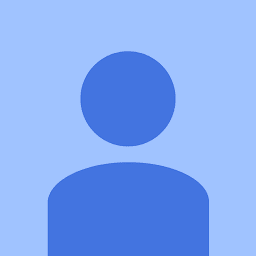
Theo Bouwman
Updated on December 30, 2021Comments
-
Theo Bouwman over 2 years
How can I programmatically close a Flutter application. I've tried popping the only screen but that results in a black screen.
-
Theo Bouwman almost 7 yearsNothing happens on both Android and iOS. Using
exit(0);
does work -
Collin Jackson almost 7 yearsOn iOS,
SystemNavigator.pop
is ignored because Apple's human interface guidelines state that applications should not exit themselves. On Android, it should work, and is preferred over callingdart:io
's exit method. Consider filing an issue: github.com/flutter/flutter/issues/new -
Ricardo Gonçalves almost 6 yearsthat didn
t work for me... I
m trying to add an exit button to CupertinoTabScafold. With ()=>exit(0), it gives a blank screen with tabbar. -
Dmytro Rostopira over 5 yearsIt works. But Apple prohibits custom exit buttons and will probably reject app if you have one
-
iPatel over 5 yearsAny idea for iOS how can we do in flutter ?
-
CopsOnRoad over 5 years@iPatel You can use exit(0) for iOS, that's it.
-
iPatel over 5 yearsYo but what about rejection ;( as you told in above comment
-
CopsOnRoad over 5 yearsYou seems like an experienced iOS developer but that question from you surprised me. Actually you should neither let your user nor you should exit the app in iOS (weird). Apple will ban your app if you try to do so. Which is why I love Android :)
-
iPatel over 5 years@CopsOnRoad Sorry for silly question I know that apple will reject the App. Actually i'm looking for Flutter but can't find any code for iOS, Above ans working in android perfectly. BTW thanks for your time and suggestion.
-
CopsOnRoad over 5 years@iPatel Don't be sorry, it's no harm to ask question. And yes, don't use exit in your app for iOS part.
-
BambinoUA over 4 yearsYou wrote so many clever notes but... what the correct way to programmatically exit from app without potential suspension in iOS as described below?
-
BambinoUA over 4 yearsIt looks like
SystemNavigator.pop()
is not working under Android emulator. The app is still working in background. So I am not sure if it will be working properly on real device. -
CopsOnRoad over 4 years@AleksTi It simply exits the app, it doesn't shut the VM process. You may try
exit(0)
for your need. -
user_odoo over 4 years@HasanAYousef In my solution get message Undefined name SystemChannels
-
Jorge Luis over 3 years
SystemNavigator.pop()
exits my app and shows a message of crash,exit(0)
works as expected -
Csaba Toth about 3 yearsWhy
SystemChannels.platform.invokeMethod('SystemNavigator.pop')
and not simplySystemNavigator.pop();
? -
Blasanka about 3 years@CsabaToth both are okay, using
invokeMethod
we are directly calling native androidpop
method. At that time Im writing may be flutter methodpop
did not exist. may be theSystemNavigator.pop();
invoke native method to exit (Check the method source code, I havent checked it). -
B.shruti almost 3 yearsIs there a way, which works for both platforms and is recommended as well ?
-
CopsOnRoad almost 3 years@B.shruti There's no way to make an exit functionality in iOS. For Android, use
SystemNavigator.pop(...)
-
tim-montague over 2 years"exit(0): Works but Apple may SUSPEND YOUR APP" - where does Apple state that in the guidelines? Please provide a link for this claim.
-
tim-montague over 2 years@CopsOnRoad - Where does Apple Human Guidelines state that apps can not exit programmatically?
-
CopsOnRoad over 2 years
-
tim-montague over 2 years@CopsOnRoad - My comment uses the word "may" - so, no argument there. Thanks for providing these links, I've edited your answer to include the archived document from Apple, but curious to know if recent Apple Guidelines still take this stance.
-
tim-montague over 2 years@CopsOnRoad - The advice you give @iPatel in these comments, does not reflect the advice you gave me (in your answer, on this question thread). From the links you shared with me, the best I can gather is that ten years ago Apple discouraged the use of
exit
, and encouraged the use of showing the user a dialog so they could close the app if they wanted to do so. However, I do not know if this is Apple's current stance, as I'm unable to find any official mention of that in Apple's iOS guidelines.