Hashmap holding different data types as values for instance Integer, String and Object
Solution 1
If you don't have Your own Data Class, then you can design your map as follows
Map<Integer, Object> map=new HashMap<Integer, Object>();
Here don't forget to use "instanceof" operator while retrieving the values from MAP.
If you have your own Data class then then you can design your map as follows
Map<Integer, YourClassName> map=new HashMap<Integer, YourClassName>();
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class HashMapTest {
public static void main(String[] args) {
Map<Integer,Demo> map=new HashMap<Integer, Demo>();
Demo d1= new Demo(1,"hi",new Date(),1,1);
Demo d2= new Demo(2,"this",new Date(),2,1);
Demo d3= new Demo(3,"is",new Date(),3,1);
Demo d4= new Demo(4,"mytest",new Date(),4,1);
//adding values to map
map.put(d1.getKey(), d1);
map.put(d2.getKey(), d2);
map.put(d3.getKey(), d3);
map.put(d4.getKey(), d4);
//retrieving values from map
Set<Integer> keySet= map.keySet();
for(int i:keySet){
System.out.println(map.get(i));
}
//searching key on map
System.out.println(map.containsKey(d1.getKey()));
//searching value on map
System.out.println(map.containsValue(d1));
}
}
class Demo{
private int key;
private String message;
private Date time;
private int count;
private int version;
public Demo(int key,String message, Date time, int count, int version){
this.key=key;
this.message = message;
this.time = time;
this.count = count;
this.version = version;
}
public String getMessage() {
return message;
}
public Date getTime() {
return time;
}
public int getCount() {
return count;
}
public int getVersion() {
return version;
}
public int getKey() {
return key;
}
@Override
public String toString() {
return "Demo [message=" + message + ", time=" + time
+ ", count=" + count + ", version=" + version + "]";
}
}
Solution 2
You have some variables that are different types in Java language like that:
message of type string
timestamp of type time
count of type integer
version of type integer
If you use a HashMap like:
HashMap<String,Object> yourHash = new HashMap<String,Object>();
yourHash.put("message","message");
yourHash.put("timestamp",timestamp);
yourHash.put("count ",count);
yourHash.put("version ",version);
If you want to use the yourHash:
for(String key : yourHash.keySet()){
String message = (String) yourHash.get(key);
Datetime timestamp= (Datetime) yourHash.get(key);
int timestamp= (int) yourHash.get(key);
}
Solution 3
Define a class to store your data first
public class YourDataClass {
private String messageType;
private Timestamp timestamp;
private int count;
private int version;
// your get/setters
...........
}
And then initialize your map:
Map<Integer, YourDataClass> map = new HashMap<Integer, YourDataClass>();
Solution 4
Create an object holding following properties with an appropriate name.
- message
- timestamp
- count
- version
and use this as a value in your map.
Also consider overriding the equals() and hashCode() method accordingly if you do not want object equality to be used for comparison (e.g. when inserting values into your map).
Solution 5
Do simply like below....
HashMap<String,Object> yourHash = new HashMap<String,Object>();
yourHash.put(yourKey+"message","message");
yourHash.put(yourKey+"timestamp",timestamp);
yourHash.put(yourKey+"count ",count);
yourHash.put(yourKey+"version ",version);
typecast the value while getting back. For ex:
int count = Integer.parseInt(yourHash.get(yourKey+"count"));
//or
int count = Integer.valueOf(yourHash.get(yourKey+"count"));
//or
int count = (Integer)yourHash.get(yourKey+"count"); //or (int)
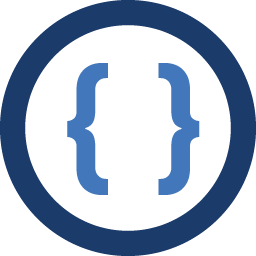
Admin
Updated on February 06, 2020Comments
-
Admin over 4 years
I need to create a hashmap with key as integer and it should hold multiple values of different data types. For example if the key is msg id and the values are
- message of type string
- timestamp of type time
- count of type integer
- version of type integer
Then how to store the values of different data type with a single key into the hashmap?
-
jlordo over 11 yearsGood suggestion, but
equals()
andhashCode()
are not important, as his keys will beInteger
. -
Will over 11 yearsAgree, not necessary for using it as a value, but I guess he might want to compare the values once retrieved from the map. And second it's good practice. I would do it in any case.
-
jlordo over 11 yearsI agree that it's good practice and upvoted your answer. But we have no clue what OP wants to do with those objects once he reads them from the map.
-
Duncan Jones over 11 years+1 for the first correct answer, but Drogba's answer is easier to read.
-
Duncan Jones over 11 yearsI also agree with @jlordo. If you draw attention to particular methods (e.g.
equals()
), you need to explain why this is important. Merely highlighting best practice will tend to bloat answers unnecessarily. -
Admin over 11 yearscan u plz provide me with an example for creating the hashmap like below and adding and searching and retrieving values from it "Map<Integer, YourClassName> map=new HashMap<Integer, YourClassName>();"
-
Rakesh Mahapatro over 11 yearsYep Sure.. please find the edited answer.. The example is only all about how to add,search, retrieve values in HashMap.
-
Merlevede over 8 yearsAlthough correct, this answer defeats the whole purpose of the OPs question. The goal of putting these values into a Map object is precisely avoiding creating tons of classes, and having the flexibility of adding as many entries in the map as needed.
-
magulla about 8 yearsequals and hashcode exist for objects to be used in collection framework, not for "I guess he might want to compare the values" ! all your answers has wrong reasoning. you can compare object with out equals and hashcode, but you cann't use them with collections and maps in proper way with out equals and hashcode !
-
Will about 8 yearsVery important point. Embarrassing how I missed this. Thanks for input. Correction made.
-
john ktejik over 6 yearsMeh. It answers the question as asked.
-
Litchy over 2 yearsThis should be a better option than casting I think.
-
Collin Fox over 2 yearsI didn't think to try casting to the datatypes, because the error I was seeing was "cannot cast type". Then I casted them, even though it said it "cannot cast type", and it worked. :')