How can I check if some checkboxes in HTML are checked?
Each check box is unique and so is uniquely named in the form.
The values themselves can be accessed via the request.form
dictionary using the name from the form as the key. For your form the checkboxes are named name1
, name2
, and name3
. But note that they are only available if they were checked on the form, otherwise their values are not posted to the server.
So in your view function you can access them like this:
name1 = request.form.get('name1')
if name1:
do_something(name1)
name2 = request.form.get('name2')
if name2:
do_something(name2)
name3 = request.form.get('name3')
if name3:
do_something(name3)
This uses get()
to perform the lookup in the form dictionary. If the key is present in the dictionary the value will be returned. If the key is not in the dictionary the value None
is returned.
As suggested by @Kevin Guan this can be handled in a for loop:
for checkbox in 'name1', 'name2', 'name3':
value = request.form.get(checkbox):
if value:
do_something(value)
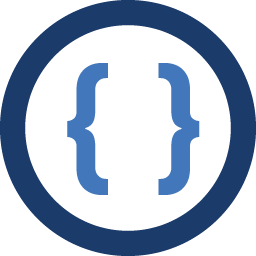
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
In am making a webpage in flask on which users can choose between several options. Depending on these options I want to show them their choosen options in the next page. My HTML code:
<input type="checkbox" name="name1">name1<br> <input type="checkbox" name="name2">name2<br> <input type="checkbox" name="name3">name3<br>
My Python code:
if checkbox 1 is checked: do something if checkbox 2 is checked: do something different if checkbox 3 is checked: do another something different
I cannot find how to form these if-statements. I found something with:
.getvalue
But then I got an error. With radio-buttons I can get it to work. When I name them all the same and give them different values with:
option = request.form['name']
However, this does also not work with checkboxes, as then it only remembers the last checkbox, but I want them all.
-
Remi Guan about 8 yearsSuggestion: Put
name1
andname2
andname3
into a list and usefor
loop instead of these threeif
statements. -
mhawke about 8 years@KevinGuan: good suggestion, thanks.