use many submit buttons in the same form
Solution 1
It won't work the way you've written it. Only the submit button you send will be included in request.form
, you'll get an error if you try to use the name of one of the other buttons.
Also, request.form.get
is a function, not a dictionary. You can use request.form.get("Histogram")
-- this will return the value of the Histogram
button if it was used, otherwise it will return None
.
Instead of giving the buttons different names, use the same name but different values.
<form id="package_form" action="" method="post">
<div class="panel-body">
<input type ="submit" name="action" value="Download">
</div>
<div class="panel-body">
<input type ="submit" name="action" value="Histogram">
</div>
<div class="panel-body">
<input type ="submit" name="action" value="Search">
</div>
</form>
Then your Python code can be:
if request.form['action'] == 'Download':
...
elif request.form['action'] == 'Histogram':
...
elif request.form['action'] == 'Search':
...
else:
... // Report bad parameter
Solution 2
Although using the same name for different submits can work (as suggested in the previous answer), it can be not so convenient for i18n.
You can still use different names, but you should check handle them differently:
if 'Download' in request.form:
...
elif 'Histogram' in request.form:
...
elif 'Search' in request.form:
...
else:
... // Report bad parameter
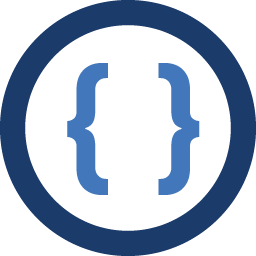
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I cannot access all the buttons from the frame. It is works only with the Histogram button.Here is my form which I want to access it in the Post method.
<form id="package_form" action="" method="post"> <div class="panel-body"> <input type ="submit" name="Download" value="Download"> </div> <div class="panel-body"> <input type ="submit" name="Histogram" value="Histogram"> </div> <div class="panel-body"> <input type ="submit" name="Search" value="Search"> </div> </form>
Here is my python code.
if request.method == 'GET': return render_template("preview.html", link=link1) elif request.method == 'POST': if request.form['Histogram'] == 'Histogram': gray_img = cv2.imread(link2,cv2.IMREAD_GRAYSCALE) cv2.imshow('GoldenGate', gray_img) hist = cv2.calcHist([gray_img], [0], None, [256], [0, 256]) plt.hist(gray_img.ravel(), 256, [0, 256]) plt.xlabel('Pixel Intensity Values') plt.ylabel('Frequency') plt.title('Histogram for gray scale picture') plt.show() return render_template("preview.html", link=link1) elif request.form.get['Download'] == 'Download': response = make_response(link2) response.headers["Content-Disposition"] = "attachment; filename=link.txt" return response elif request.form.get['Search'] == 'Search': return link1
What I am doing wrong?
-
jlplenio over 3 yearsMy request.form had no post attribute. Found the actions in form itself.
-
Barmar over 2 yearsDoes your form have an input with
name="action"
?