How can I get current base URI in flask?
38,783
Solution 1
You can use the base_url
method on flask's request
function.
from flask import Flask, request
app = Flask(__name__)
@app.route('/foo')
def index():
return request.base_url
if __name__ == '__main__':
app.run()
This returns the following if the app route is /foo
:
http://localhost:5000/foo
Solution 2
Use flask.request.url
to retrieve your requested url. Have a look at: http://flask.pocoo.org/docs/1.0/api/#flask.Request (or the v0.12 docs)
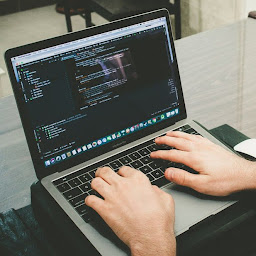
Author by
Ravi Bhushan
Updated on July 24, 2020Comments
-
Ravi Bhushan almost 4 years
In below code, i want to store URL in a variable to check error on which URL error occured.
@app.route('/flights', methods=['GET']) def get_flight(): flight_data= mongo.db.flight_details info = [] for index in flight_data.find(): info.append({'flight_name': index['flight_name'], 'flight_no': index['flight_no'], 'total_seat': index['total_seat'] }) if request.headers['Accept'] == 'application/xml': template = render_template('data.xml', info=info) xml_response = make_response(template) xml_response.headers['Accept'] = 'application/xml' logger.info('sucessful got data') return xml_response elif request.headers['Accept'] == 'application/json': logger.info('sucessful got data') return jsonify(info)
Output:
* Restarting with stat * Debugger is active! * Debugger PIN: 165-678-508 * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit) 127.0.0.1 - - [28/Mar/2017 10:44:53] "GET /flights HTTP/1.1" 200 -
I want this message
"127.0.0.1 - - [28/Mar/2017 10:44:53] "GET /flights HTTP/1.1" 200 -"
should be stored in a variable or how can I get current URL that is executing?
-
Ravi Bhushan about 7 years127.0.0.1 - - [28/Mar/2017 10:44:53] "GET /flights HTTP/1.1" 200 -... if want to return whole thing , then what have i to do ?
-
Neill Herbst about 7 years@RaviBhushan I'm not aware of any method to capture the standard output of the flask server.
-
Ravi Bhushan about 7 yearsok.... thanx for answer