How do you convert variable names to a string in Dart?
Solution 1
There is no way in Dart to get an objects name. C# for instance has the nameof()
function, but Dart has nothing like it.
That being said there is probably a much easier way of doing whatever your reason for needing this is. So feel free to ask for alternative approaches.
If you just want to use the variable name as an identifier of sorts, maybe look into Maps.
Map<String,String> x = {"ctrB" : "b"};
They let you define a key and value of whatever type you want.
EDIT: as an aside, this declaration
class ctr {
String i;
ctr(String a){
this.a = a;
}
can be simplified to this:
class ctr {
String i;
ctr(this.i);
}
Solution 2
This is how you should declare a list of custom object.
List<ctr> ctrList= [ ctrA , ctrB , ctrC ];
void main() {
String match = 'ctrB';
for (var index = 0; i<ctrList.length; i++) {
if(match == ctrList[index].i {
print('The string of your match is ${ctrList[i].a}');
} else {
print('Error!);
}}
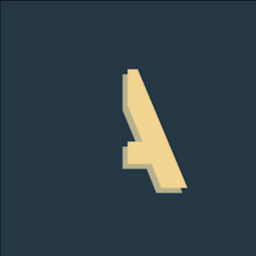
ndisisnd
Updated on December 21, 2022Comments
-
ndisisnd over 1 year
Very new to Flutter and Dart, so pardon me for the ill-phrased question.
This is a generic code that I have:
// Constructor class class ctr { String i; ctr(String a){ this.a = a; } // New variables based on contsructor var ctrA = ctr('a'); var ctrB = ctr('b'); var ctrC = ctr('c'); // Storing the variables based on constructor in an array List ctrList = [ctrA, ctrB, ctrC] void main() { String match = 'ctrB'; for (var i = 0; i<ctrList.length; i++) { if(match == ctrList.toString() { print('The string of your match is ${ctrList[i].a}'); } else { print('Error!); } } }
The current output is
Error!
. Instead, what I'm looking for isa
.As you can see, I'm trying to iterate the variable
match
over the array that stores the variables based on an aforementioned constructor, and if it matches, print out the value in the constructor that it has matched to.runtimeType
can only get me the name of the origin Class (which will bectr
).I tried to convert the variable name by using
toString()
but that didn't work either. What I need is a method to convert these variable names to a string and get a match, but I'm not sure what I can do.Thanks in advance for the help!
-
Hritik Gupta almost 4 yearsFollow CamelCase convention while declaring class name.
-
-
ndisisnd almost 4 yearsWhen the element type is Map<String, String>, what's the element type then when it's stored in the array? I assume my List<ctr> can't work anymore.
-
Benedikt J Schlegel almost 4 yearsIt would be List<Map<String,String>> so a list of maps of strings :D. Of course you can still keep your object if you want, by using Map<String,ctr> instead, or any other combination of types.