How to create pdf file and send in mail of spring boot application
This is how you can send a mail:
public void email() {
String smtpHost = "yourhost.com"; //replace this with a valid host
int smtpPort = 587; //replace this with a valid port
String sender = "[email protected]"; //replace this with a valid sender email address
String recipient = "[email protected]"; //replace this with a valid recipient email address
String content = "dummy content"; //this will be the text of the email
String subject = "dummy subject"; //this will be the subject of the email
Properties properties = new Properties();
properties.put("mail.smtp.host", smtpHost);
properties.put("mail.smtp.port", smtpPort);
Session session = Session.getDefaultInstance(properties, null);
ByteArrayOutputStream outputStream = null;
try {
//construct the text body part
MimeBodyPart textBodyPart = new MimeBodyPart();
textBodyPart.setText(content);
//now write the PDF content to the output stream
outputStream = new ByteArrayOutputStream();
writePdf(outputStream);
byte[] bytes = outputStream.toByteArray();
//construct the pdf body part
DataSource dataSource = new ByteArrayDataSource(bytes, "application/pdf");
MimeBodyPart pdfBodyPart = new MimeBodyPart();
pdfBodyPart.setDataHandler(new DataHandler(dataSource));
pdfBodyPart.setFileName("test.pdf");
//construct the mime multi part
MimeMultipart mimeMultipart = new MimeMultipart();
mimeMultipart.addBodyPart(textBodyPart);
mimeMultipart.addBodyPart(pdfBodyPart);
//create the sender/recipient addresses
InternetAddress iaSender = new InternetAddress(sender);
InternetAddress iaRecipient = new InternetAddress(recipient);
//construct the mime message
MimeMessage mimeMessage = new MimeMessage(session);
mimeMessage.setSender(iaSender);
mimeMessage.setSubject(subject);
mimeMessage.setRecipient(Message.RecipientType.TO, iaRecipient);
mimeMessage.setContent(mimeMultipart);
//send off the email
Transport.send(mimeMessage);
System.out.println("sent from " + sender +
", to " + recipient +
"; server = " + smtpHost + ", port = " + smtpPort);
} catch(Exception ex) {
ex.printStackTrace();
} finally {
//clean off
if(null != outputStream) {
try { outputStream.close(); outputStream = null; }
catch(Exception ex) { }
}
}
}
You can see that we create a MimeBodyPart
with a DataSource
created from bytes
that resulted from a method named writePdf()
:
public void writePdf(OutputStream outputStream) throws Exception {
Document document = new Document();
PdfWriter.getInstance(document, outputStream);
document.open();
Paragraph paragraph = new Paragraph();
paragraph.add(new Chunk("hello!"));
document.add(paragraph);
document.close();
}
Since we use a ByteOutputStream
instead of a FileOutputStream
no file is written to disk.
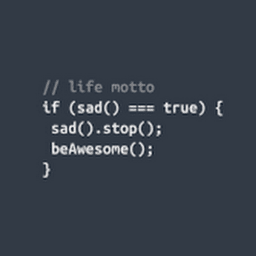
learn program
Updated on June 28, 2022Comments
-
learn program almost 2 years
I am trying to send email with created pdf document as the attachment, the environment i am working is the REST based java spring boot application,
Actually i know how to send email with the thymeleaf template engine, but how can i create a pdf document in memory, and send it as a attachment, this is the code that i am using for sending email.
Context cxt = new Context(); cxt.setVariable("doctorFullName", doctorFullName); String message = templateEngine.process(mailTemplate, cxt); emailService.sendMail(MAIL_FROM, TO_EMAIL,"Subject", "message");
and this this the sendmail() function
@Override public void sendPdfMail(String fromEmail, String recipientMailId, String subject, String body) { logger.info("--in the function of sendMail"); final MimeMessage mimeMessage = this.mailSender.createMimeMessage(); try { final MimeMessageHelper message = new MimeMessageHelper(mimeMessage, true, "UTF-8"); message.setSubject(subject); message.setFrom(fromEmail); message.setTo(recipientMailId); message.setText(body, true); FileSystemResource file = new FileSystemResource("C:\\xampp\\htdocs\\project-name\\logs\\health360-logging.log"); message.addAttachment(file.getFilename(), file); this.mailSender.send(mimeMessage); logger.info("--Mail Sent Successfully"); } catch (MessagingException e) { logger.info("--Mail Sent failed ---> " + e.getMessage()); throw new RuntimeException(e.getMessage()); } }
Actually i need to create a kind of report with 2-3 pages as pdf file, and send in mail.
Also i need to send multiple pdf reports, in the mail, how can i do this, can you friends help me on this, i found something called jasper, is it something related to my environment,
-
learn program almost 7 yearsWhat is the Document class here, javax.swing.text or org.dom4j or org.thymeleaf.dom or org.w3c.dom
-
Bruno Lowagie almost 7 yearsIt's a
com.itextpdf.text.Document
object in iText 5. If you want the most recent version, check out the iText 7 documentation: developers.itextpdf.com/content/itext-7-jump-start-tutorial -
Pawan Tiwari almost 5 yearsiText is paid now.? Is there any alternative of itext apart from jasper
-
Eva M over 2 yearsYes @PawanTiwari, you can use OpenPdf ! It is a fork of iText and shares many of its concepts and classes.