How to download image from ip camera in Flutter
1,240
How about instead of image downloader use
import 'package:http/http.dart' as http;
import 'dart:io';
import 'package:path_provider/path_provider.dart';
import 'package:flutter/foundation.dart';
Future<File> _downloadFile(String authEncoded,String url, String param) async {
final response = await http.post(url,
headers: {
HttpHeaders.authorizationHeader: 'Basic ' + authEncoded
// Do same with your authentication requirement
},
body: param,
);
response.headers.forEach((k,v) => print (k+" : "+v));
//Your response headers here
if(response.statusCode==200){
String dir = (await getApplicationDocumentsDirectory()).path;
File file = new File('$dir/image.jpg');
await file.writeAsBytes(response.bodyBytes);
return file;
} else {
print("Error : " + response.statusCode.toString());
}
}
Now Call
File file = await _downloadFile("http://192.168.1.102:13237/snapshot.cgi", "<GetSnapshotUri xmlns=\"http://www.onvif.org/ver20/media/wsdl\"><ProfileToken>PROFILE_000</ProfileToken></GetSnapshotUri>");
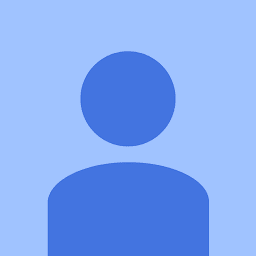
Author by
Стас Фролкин
Updated on December 16, 2022Comments
-
Стас Фролкин over 1 year
When I send http request to Ip camera (Onvif)
"<GetSnapshotUri xmlns=\"http://www.onvif.org/ver20/media/wsdl\">" + "<ProfileToken>PROFILE_000</ProfileToken></GetSnapshotUri>";
I got Response containing Url:
.... <s:Body><trt:GetSnapshotUriResponse><trt:MediaUri> <tt:Uri>http://192.168.1.102:13237/snapshot.cgi</tt:Uri> <tt:InvalidAfterConnect>false</tt:InvalidAfterConnect> <tt:InvalidAfterReboot>false</tt:InvalidAfterReboot><tt:Timeout>PT5S</tt:Timeout></trt:MediaUri> </trt:GetSnapshotUriResponse></s:Body></s:Envelope>
I've tried to use image_downloader plugin, not worked. I can see it in browser(Chrome) but I can't view it via Image.network('http://192.168.1.102:13237/snapshot.cgi') Widget
I got error
════════ Exception caught by image resource service ════════════════════════════════════════════════ The following NetworkImageLoadException was thrown resolving an image codec: HTTP request failed, statusCode: 401, http://192.168.1.102:13237/snapshot.cgi When the exception was thrown, this was the stack: #0 NetworkImage._loadAsync (package:flutter/src/painting/_network_image_io.dart:90:9) <asynchronous suspension> #1 NetworkImage.load (package:flutter/src/painting/_network_image_io.dart:47:14) #2 ImageProvider.resolve.<anonymous closure>.<anonymous closure>.<anonymous closure> (package:flutter/src/painting/image_provider.dart:327:17) #3 ImageCache.putIfAbsent (package:flutter/src/painting/image_cache.dart:160:22) ... Image provider: NetworkImage("http://192.168.1.102:13237/snapshot.cgi", scale: 1.0) Image key: NetworkImage("http://192.168.1.102:13237/snapshot.cgi", scale: 1.0) ════════════════════════════════════════════════════════════════════════════════════════════════════
I've found answer on Java
URL url = new URL("http://webacm-ip-adr:8084/snapshot.cgi"); InputStream input = url.openStream(); String jpg = "sample.jpg"; FileOutputStream output = new FileOutputStream(jpg); IOUtils.copy(input, output);
Updated after get correct answer. I can see params needed for create Digest key, but due I can't return Response object by dart code Response header in http sniffer that I can't get by Dart code
-
Стас Фролкин over 4 yearsThanks for answer. I get error: Unhandled Exception: FileSystemException: Cannot open file, path = 'image.jpg' (OS Error: Read-only file system, errno = 30)
-
Стас Фролкин over 4 yearsHTTP/1.1 401 Unauthorized Server: GoAhead-Webs Date: Thu Dec 26 05:51:48 2019 WWW-Authenticate: Digest realm="goAhead", domain=":31122",qop="auth", nonce="cdb3f5e3d9fe4fc5768726b5920f97ba", opaque="5ccc069c403ebaf9f0171e9517f40e41",algorithm="MD5", stale="FALSE" Pragma: no-cache Cache-Control: no-cache Content-Type: text/html <html><head><title>Document Error: Unauthorized</title></head> <body><h2>Access Error: Unauthorized</h2> <p>Access to this document requires a User ID</p></body></html>
-
Dev over 4 yearsis there any requirement of basic auth to access the url?
-
Стас Фролкин over 4 yearsYour answer is correct, I just need to pass one more auth step for getting snapshot
-
Стас Фролкин over 4 yearsCould you give me a hint what is easiest way to pass digest auth on Dart. include params like realm, nonce and etc
-
Стас Фролкин over 4 yearsHow can I get header of response for extract params for digest generate? final response = await http.post(url, headers: { }, body: param, ); after that code I get nothing by expression print(response.headers);
-
Dev over 4 yearsi have set an example for basic auth similarly you can set digest in header
-
Стас Фролкин over 4 yearsYes understand that. Why I can't return Response object when I do request to this url = 192.168.88.32:31122/snapshot.cgi. I only see realm, nonce and etc in http sniffer. When I do request to url = 192.168.88.32:10080. There's no problem to get Response object
-
Dev over 4 yearsReally cant help beyond this as don't know your exact setup and what request response are being played. Try to debug headers, responses, etc by printing them.
-
Стас Фролкин over 4 yearsThere is a Response object in your code. So Ip camera Response with 401 code Unauthorized (I've added screenshot of sniffer at the end of Question), but Why I can't get this error from Response object in your code? I can't print nothing from Response object
-
Dev over 4 yearsalso added values of response headers
-
Стас Фролкин over 4 yearsCould you try to help with my next authentification problem stackoverflow.com/questions/59491178/…