How to extract result content from play.mvc.Result object in play application?
Solution 1
Use JavaResultExtractor, example:
Result result = ...;
byte[] body = JavaResultExtractor.getBody(result, 0L);
Having a byte array, you can extract charset from Content-Type header and create java.lang.String:
String header = JavaResultExtractor.getHeaders(result).get("Content-Type");
String charset = "utf-8";
if(header != null && header.contains("; charset=")){
charset = header.substring(header.indexOf("; charset=") + 10, header.length()).trim();
}
String bodyStr = new String(body, charset);
JsonNode bodyJson = Json.parse(bodyStr);
Some of above code was copied from play.test.Helpers
Solution 2
I think play.test.Helpers.contentAsString
is what you're looking for.
public static java.lang.String contentAsString(Result result)
Extracts the content as String.
Still available in Play 2.8.x.
Solution 3
This functions works fine for me.. Thanks to Mon Calamari
public static JsonNode resultToJsonNode(Result result) {
byte[] body = JavaResultExtractor.getBody(result, 0L);
ObjectMapper om = new ObjectMapper();
final ObjectReader reader = om.reader();
JsonNode newNode = null;
try {
newNode = reader.readTree(new ByteArrayInputStream(body));
Logger.info("Result Body in JsonNode:" + newNode.toString());
} catch (JsonProcessingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return newNode;
}
}
Solution 4
You'll need to pass an instance of akka.stream.Materializer into JavaResultExtractor's getbody method.
Use Google Guice for Injecting at constructor level or declaration level.
@Inject
Materializer materializer;
and Further you can convert Result into String or any other type as required:
Result result = getResult(); // calling some method returning result
ByteString body = JavaResultExtractor.getBody(result, 1, materializer);
String stringBody = body.utf8String(); // get body as String.
JsonNode jsonNodeBody = play.libs.Json.parse(stringBody); // get body as JsonNode.
Solution 5
redirect
return results with error code 303, which cause the caller (browser) to do another request to the given url.
What you need to do is actually proxying. Application1 should make a request to Application2, and handle the response.
Play have very nice Web Service API which allow doing this easily.
Related videos on Youtube
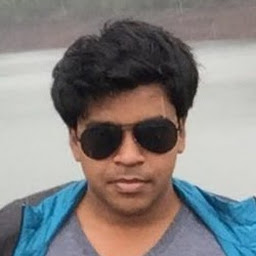
Jayaprakash Narayanan
Updated on October 27, 2022Comments
-
Jayaprakash Narayanan less than a minute
actually I am doing redirect from one play application to another play application, finally I receive response as Result object.. Below is the action in two applications. I am redirecting from apllication1 to application2. Application 2 will return JSON string, that I need to extract.
How can I retrieve JSON content from Result object?
Application1:
public static Result redirectTest(){ Result result = redirect("http://ipaddress:9000/authenticate"); /*** here I would like to extract JSON string from result***/ return result; }
Application2:
@SecuredAction public static Result index() { Map<String, String> response = new HashMap<String, String>(); DemoUser user = (DemoUser) ctx().args.get(SecureSocial.USER_KEY); for(BasicProfile basicProfile: user.identities){ response.put("name", basicProfile.firstName().get()); response.put("emailId", basicProfile.email().get()); response.put("providerId", basicProfile.providerId()); response.put("avatarurl", basicProfile.avatarUrl().get()); } return ok(new JSONObject(response).toString()); }
-
Sanjeev Kumar Dangi about 6 yearsJavaResultExtractor.getBody is giving timeoutexception, even if i increased value from 0 to 100k.
-
Sanjeev Kumar Dangi about 6 yearsJavaResultExtractor.getBody(result, 0L) gives timeout even if i change it from 0 to 100k
-
Fahad over 5 yearsWondering why don't use play.test.Helpers.contentAsString(result) ?
-
Mon Calamari over 5 yearsHelpers class have test scope.
-
Dirk Hillbrecht almost 5 yearsAfter one and a half days of searching, this was the only place where I found this solution for "creating" a "Materializer" in Play-2.5-context. This should really have been mentioned in the migration guide from Play 2.4 to Play 2.5...