How to have Java method return generic list of any type?
209,315
Solution 1
private Object actuallyT;
public <T> List<T> magicalListGetter(Class<T> klazz) {
List<T> list = new ArrayList<>();
list.add(klazz.cast(actuallyT));
try {
list.add(klazz.getConstructor().newInstance()); // If default constructor
} ...
return list;
}
One can give a generic type parameter to a method too. You have correctly deduced that one needs the correct class instance, to create things (klazz.getConstructor().newInstance()
).
Solution 2
No need to even pass the class:
public <T> List<T> magicalListGetter() {
return new ArrayList<T>();
}
Solution 3
Another option is doing the following:
public class UserList extends List<User>{
}
public <T> T magicalListGetter(Class<T> clazz) {
List<?> list = doMagicalVooDooHere();
return (T)list;
}
List<User> users = magicalListGetter(UserList.class);
`
Solution 4
Let us have List<Object> objectList
which we want to cast to List<T>
public <T> List<T> list(Class<T> c, List<Object> objectList){
List<T> list = new ArrayList<>();
for (Object o : objectList){
T t = c.cast(o);
list.add(t);
}
return list;
}
Solution 5
Something like this
publiс <T> List<T> magicalListGetter(Class<T> clazz) {
List list = doMagicalVooDooHere();
return list;
}
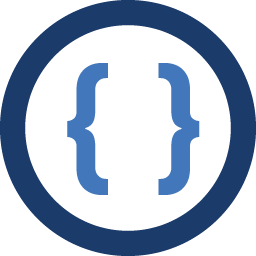
Author by
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I would like to write a method that would return a
java.util.List
of any type without the need to typecast anything:List<User> users = magicalListGetter(User.class); List<Vehicle> vehicles = magicalListGetter(Vehicle.class); List<String> strings = magicalListGetter(String.class);
What would the method signature look like? Something like this, perhaps(?):
public List<<?> ?> magicalListGetter(Class<?> clazz) { List<?> list = doMagicalVooDooHere(); return list; }
-
newacct almost 11 yearswhy pass
clazz
if you're not gonna use it? -
Evgeniy Dorofeev almost 11 yearsit was like this in the question, probably OP is going to use it somehow
-
zygimantus over 6 yearsI think that you need to pass a class when you want to add items.
-
bhlowe over 4 yearsThis is the best solution if you want to manipulate the list and simply return the same type. (For instance, reversing the order, reducing the number of items, etc.) Passing a class would only be needed if you need to instantiate a new object of type T.