importing a variable from one typescript file into another
Solution 1
The best way to handle server api urls is to use the angular environment files. There is two advantages to use it:
- Available in all your application
- You can handle multiples platform (localhost, dev, stating, prod) without modifing your code
in app/environments
you can create differents files:
- environments.prod.ts
- environments.ts
- environement.test.ts
In each files you defines your gobals variables:
for localhost:
export const environment = {
production: false,
apiHost: 'http://localhost',
recaptchaKey: '6LeWzxQUeazeAAPpR0gZFezaeazL5AvUP3moN1U4u',
fileHost: 'http://file.localhost',
};
for prod example:
export const environment = {
production: true,
apiHost: 'http://prod',
recaptchaKey: '6LeWzxQUeazeAAPpR0gZFezaeazL5AvUP3moN1U4u',
fileHost: 'http://file.prod',
};
To use it in your scripts you only need to always import
import { environment } from './environments/environment'; //relative path from where your file is
import { environment } from './environments/environment'
export class Service {
protected cityListUrl = '/api/city/';
constructor(protected http: Http) { }
get() {
this.http.get(environment.apiHost + this.cityListUrl).map(response => response.json());
}
}
It's when you build your project with angular-cli, you precise which environment you want to use
ng build --environment=prod
or
ng serve --environment=test
Which it is cool because you can easly integrate this command line in a continuous integration tools.
Solution 2
The easiest way would be to just define and export each variable independently. This way you can also import them independently as well.
export const rooturl = 'http://127.0.0.1:8000';
export const cityListUrl = rooturl + '/api/city/'
And import them this way.
import {Injectable} from '@angular/core';
import {Http} from '@angular/http';
import {cityListUrl} from 'app/backendUrls';
@Injectable()
export class cityGetService{
constructor(private http: Http){}
cityGet(){
this.http.get(cityListUrl)
}
}
If you need them all together in an object just export them like that as well.
export const rooturl = 'http://127.0.0.1:8000';
export const cityListUrl = rooturl + '/api/city/'
export const all = {
rooturl, cityListUrl
}
The way you have it now it's a class, that must be instantiated in order to get access to it's instance properties.
The generated code for your class looks like this.
define(["require", "exports"], function (require, exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var backendUrls = (function () {
function backendUrls() {
// root url
this.rooturl = 'http://127.0.0.1:8000';
//get a list of all cities
this.cityListUrl = this.rooturl + '/api/city/';
}
return backendUrls;
}());
exports.backendUrls = backendUrls;
});
If you need a class you will first have to make an instance of it using the new keyword.
import {Injectable} from '@angular/core';
import {Http} from '@angular/http';
import {backendUrls} from 'app/backendUrls';
@Injectable()
export class cityGetService{
constructor(private http: Http){}
cityGet(){
this.http.get(new backendUrls().cityListUrl)
}
}
If you need to use a class but want to have this in a static manner you can make the properties static then they'll get defined as properties on the class itself instead of an instance.
export class backendUrls {
// root url
static rooturl: string = 'http://127.0.0.1:8000';
// need to use the class to access the root since we don't have an instance.
static cityListUrl: string = backendUrls.rooturl + '/api/city/';
}
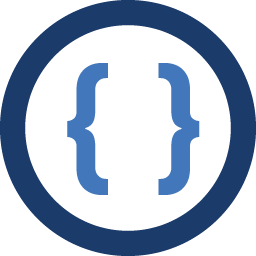
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
I am attempting to import a variable from one type script file into another.
the variable I want to import is
cityListUrl
the type script file it is in is coded like this:
export class backendUrls{ // root url rooturl:string= 'http://127.0.0.1:8000'; //get a list of all cities cityListUrl:string = this.rooturl + '/api/city/'; }
the file I want to import it into looks like this:
import {Injectable} from '@angular/core'; import {Http} from '@angular/http'; import {backendUrls} from 'app/backendUrls'; @Injectable() export class cityGetService{ constructor(private http: Http){} cityGet(){ this.http.get(backendUrls.cityListUrl) } }
the
cityListUrl
in my pycharm editor is currently red. with the messageTS2339: 'cityListUrl' does not exist on type 'typeof backendUrls'.
has anyone had this issue before? How would I fix this? Thank you
-
Admin almost 7 yearsinterestingly the ; at the end of each variable is now red with the warning expected , whats going on with that?
-
Admin almost 7 yearswell i have no more errors in the cityGetService.ts file which means your solution worked thank you. Its the silly ; , issue I commented above. I am thinking its just pycharm complaining for no reason. I'll accept your answer and thank you oh your updating yay thank you
-
toskv almost 7 yearsactually the first solution doesn't really work, since this.rootUrl is not defined because does not point to backendUrl. and cityurl will end up as "undefined/api/city/"
-
Admin almost 7 yearsdarn ok walk me through sensei
-
Admin almost 7 yearsdoes this mean I can get rid of the export const backendUrls? That the only thing I need to have in this file are the two variables with the exports on them?
-
toskv almost 7 yearsyep, you can export them all as part of an object too if you need to have them grouped like that for some reason, I can add an example for that too if you want. :)
-
Admin almost 7 yearsno thank you on that. We are close though. One more wrinkle it seems. I removed the export const backendUrls and just have the export const variables. I removed the this from the rooturl in cityListUrl I now have an error on the rooturl in cityListUrl which says: expected = and expected new line or semicolon very werid
-
Admin almost 7 yearsoops never mind got it forgot to remove the : and add a = its cause im stupid lol