Storing a HashMap in an SQL database
Solution 1
You need a 3 column table
user,
key,
value
Would then look like
"user 1", "property1", "value1"
"user 1", "property2", "value2"
"user 2", "property1", "value1"
"user 3", "property1", "value1"
"user 3", "property2", "value2"
You would then just read the data back in and look round the user field to rebuild each has table.
Solution 2
If you want to stick key-value you can, however, you've to make sure the keys and values are strings and can fit in the column definition. Here is an example:
mysql> create table hashmap (k varchar(200), v varchar(200));
Query OK, 0 rows affected (0.24 sec)
mysql> insert into hashmap values ('key','value');
Query OK, 1 row affected (0.02 sec)
mysql> select * from hashmap;
+------+-------+
| k | v |
+------+-------+
| key | value |
+------+-------+
1 row in set (0.00 sec)
Additionally, you can unique index the column k
which holds the keys so your lookups are constant just like a hashmap, and you can replace the old value by using ON DUPLICATE KEY UPDATE v = new_value.
. I am assuming you're using MySQL for the ON DUPLICATE KEY
part.
Solution 3
HashMap is data structure, You can store data residing in it.
Create a table emulating key value pair. iterate through keys of map and store it in DB.
Solution 4
Each entry in the HashMap is like a row in your table. You would end up having a separate table for each HashMap (probably).
Your K
parameter in your map should be enforced as a unique key on your table, and indexed.
Keep in mind that storing these objects may require more than one column each. For example, you may have a Point object as your key, so you'd need a column x and column y, and an index on uniqueness for x and y pairs.
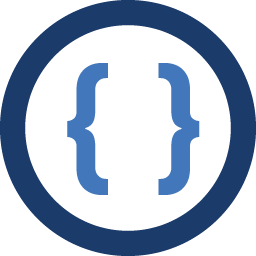
Admin
Updated on December 01, 2020Comments
-
Admin over 3 years
How do you store a HashMap inside of an SQL database? Also, how would you load that HashMap from a SQL database back into an instance of a HashMap?
Okay, this is what I'm doing. I have a database to store the player data for my game. It has a table containing their usernames and passwords. Each player has a HashMap that stores their properties. I need to store that HashMap in the database along with it's respective user.
-
Oded about 13 years@Gnarly - His point is that SQL databases are about storing data, not data structures.
-
Admin about 13 yearsYes, but I want to store the data inside of the HashMap inside of the database.
-
Admin about 13 yearsHow is this related to my question?
-
lobster1234 about 13 yearsHow is it not related to your question? You wanted to stick the data inside a hashmap in a table, and the schema I outlined does just that, except that key and value are strings. Can you add more details and possibly give an example, as from the comments its fairly obvious that no one really understands what you want answered.
-
jmj about 13 yearsYou just need to execute insert query using JDBC
-
Admin about 13 yearsI don't know anything about SQL databases, thus why I am asking how to on SO.
-
Admin about 13 yearsI was originally thinking this would be the way I'd have to do it, but I figured there was probably a better way.
-
jmj about 13 yearsquick googling turned into this
-
pgbsl about 13 yearsI think that this is a nice, simple solution. Simple and easy to read trumps clever solutions every time in my opinion. Nice and easy to maintain.
-
Admin about 13 yearsI have no idea how to implement this idea into code with the SQL framework in Java.
-
pgbsl about 13 yearsGet an entry set from the hashmap, then iterate across it. See @Jigar s comment for link to sql tutorials. There is plenty of info out there already on how to do this, that will be tailored to the database that you are using.
-
Admin about 13 yearsMy problem isn't with SQL's syntax, it's with the Java SQL framework. I spent an hour last night trying to figure out how to iterate through the entry set of the HashMap and then making an UPDATE query to the database for each entry. It wasn't working no matter what I was doing.
-
Admin about 13 yearsI would but I recently gave up and went back to XML. I still want to learn how to use the Java SQL framework effectively. If anyone could point me in the direction of a good tutorial covering all the basic parts of using it.