storing hashMap in a hashMap
Solution 1
Example:
Creating and populating the maps
Map<String, Map<String, Value>> outerMap = new HashMap<String, HashMap<String, Value>>();
Map<String, Value> innerMap = new HashMap<String, Value>();
innerMap.put("innerKey", new Value());
Storing a map
outerMap.put("key", innerMap);
Retrieving a map and its values
Map<String, Value> map = outerMap.get("key");
Value value = map.get("innerKey");
Solution 2
Creating two Simple Hashmaps: InnerMap and OuterMap
HashMap<String, HashMap<String, String>> outerMap = new HashMap<String, HashMap<String,String>>();
HashMap<String, String> innerMap = new HashMap<String, String>();
Populating the HashMaps
innerMap.put("InnerKey", "InnerValue");
outerMap.put("OuterKey", innerMap);
Retreiving values from HashMaps
String value = ((HashMap<String, String>)outerMap.get("OuterKey")).get("InnerKey").toString();
System.out.println("Retreived value is : " + value);
Solution 3
You get something that looks like a 2 dimensions HashMap, so to say. Which means you need 2 String to store a value, and also to retrieve one.
You could, for example write a class to wrap that complexity, like that (untested code):
public class HashMap2D<T> {
private HashMap<String,HashMap<String,T>> outerMap;
public HashMap2D() {
outerMap = new HashMap<String,HashMap<String,T>>();
}
public void addElement(String key1, String key2, T value) {
innerMap=outerMap.get(key1);
if (innerMap==null) {
innerMap = new HashMap<String,T>();
outerMap.put(key1,innerMap);
}
innerMap.put(key2,value);
}
public T getElement(String key1, String key2) {
Hashmap innerMap = outerMap.get(key1);
if (innerMap==null) {
return null;
}
return innerMap.get(key2);
}
}
If you want methods to process more than one data at a time, it's more complicated, but follows the same principles.
Solution 4
This will solve the same problem using one map (although, this does not directly answer your question) by flattening two nested maps into one big map, using a double-key.
public class Key2D{
private final String outer;
private final String inner;
public Key2D(String outer, String inner){
this.outer = outer;
this.inner = inner;
}
//include default implementations for
//Object.equals(Object) and Object.hashCode()
//Tip: If you're using Eclipse it can generate
//them for you.
}
Then just create one map with double-key:
Map<Key2D, Value> map = new HashMap<Key2D, Value>();
map.put(new Key2D("outerKey", "innerKey"), "Value");
map.get(new Key2D("outerKey", "innerKey")); // yields "Value"
This gives a shorter solution. Performance wise it's probably about the same. Memory performance is probably slightly better (just guessing, though).
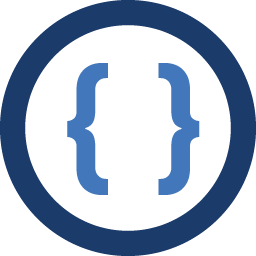
Admin
Updated on July 05, 2020Comments
-
Admin almost 4 years
i am reading data from a text file and want to store HashMap in another HashMap..
HashMap<string,HashMap<string,value>>
how to store data and retrieve it? any sample code will be appreciated... thank u
-
The Scrum Meister over 13 yearsNote: in java its
String
with a upper S. -
Trinidad over 13 yearsThere is no use to store the same instance of
innerMap
for each key inouterMap
, it would be the same as not havingouterMap
. You should create a new instance of the "inner map" for each new key inouterMap
. -
AnshulGarg over 13 years@Trinidad: Thats absolutely correct. For each new key in outermap there will be a new instance of innerMap. I was just trying to demonstrate "how to populate the value in outermap with one key, and then just retreiving its value".
-
Trinidad over 13 yearsOh, but it confuses to instantiate both maps in the same place, you should have put the creation of the new inner map instance with the code to populate it. =)
-
Snake over 11 yearsEdit: Map<String, Map<String, Value>> outerMap = new HashMap<String, Map<String, Value>>(); I was getting a type mismatch exception.
-
Sohaib over 9 years@Johan What if I need to update the inner map after putting it in the outer map? Can that be done?
-
Luke Chamberlain over 9 years@Sohaib You would have to get() the innerMap, update it then put it again.
-
Sohaib over 9 years@LukeChamberlain I don't think put() again is required since the get would return a reference. Just get() and updating it would be enough. I did some experiments yesterday and it worked.
-
Alexis Dufrenoy about 3 yearsSo you are nesting Hashmaps, but just to avoid retrieving the inner one, you recreate it each time. That's a waste of performance.