String as a key in HashMap
Solution 1
Any object that provides a meaningful implementation of hashCode()
is a perfect key candidate in a map: see Understanding the workings of equals and hashCode in a HashMap.
Also, as @Jon mentioned, all keys in your map should be of the same type.
EDIT: Of course, you need to implement both equals()
and hashcode()
. I thought the title of the link to the other question made that clear. But a dumb implementation of hashcode()
will just bring you a degenerate HashMap
which performance is poor.
EDIT2: As @Adrian mentioned in his answer, generics will help you constrain the type of keys and values for the map.
References:
- Effective Java Programming Language Guide, sample chapter about equals() and hashCode()
- Java theory and practice: Hashing it out
- Equals and HashCode
Solution 2
A raw HashMap
will indeed accept any object as key. However, it is good style to specify which kind of keys and values you are going to use in a map
Map<String, Whatever> map = new HashMap<String, Whatever>();
if you do so, the put()
method will only accept strings.
NB: if you choose to use one of your own classes as keys, make sure the class either implements both equals
and hashCode
or none of them!
Solution 3
One probable reason why you see "String" used as hash key quite often is that it is an
immutable class.
Immutable objects make great map keys since one need not worry about their values
being modified once they're in a map or set, which would destroy
map or set's invaraints (Item 15 Effective Java, 2nd Edition)
Solution 4
One of the reason why we generally use String as a key in HashMap is that since String is immutable in Java that allows String to cache its hashcode , being immutable String in Java caches its hash code and do not calculate every time we call hashcode method of String, which makes it very fast as HashMap key.
Solution 5
You haven't shown which platform you're talking about, but I'll assume for the moment that it's Java.
You can use any type for the key in a HashMap
, but assuming you use the full generic version you need to specify that type and then use it consistent. For example, you could use URI as the key type:
HashMap<URI, Integer> hitCountMap = new HashMap<URI, Integer>();
The get
method takes Object as the key parameter type, but you should of course use the same type of key that you've put into the map. See this question for more discussion on that.
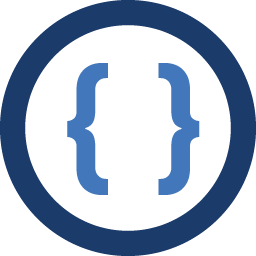
Admin
Updated on July 24, 2022Comments
-
Admin almost 2 years
I had seen, only the String is used as a key in HashMap.Although the put() method takes Object as a parameter.What is the significant of it.If any other object can also used as a Key or not? Please provide the answers.