How to use convert command with bash to resize all images in a given directory?
Here is one way (put it in a file and execute it with any POSIX shell like bash
or ksh
):
cd ~/somefolder/ || exit 1
for f in *.png
do
case $f in
(tn_*) continue ;;
(*) convert "${f}" -resize 50%x50% "tn_${f}" ;;
esac
done
With modern shells the case
construct could also be replaced by a terser conditional command:
cd ~/somefolder/ || exit 1
for f in *.png
do
[[ "$f" != tn_* ]] && convert "${f}" -resize 50%x50% "tn_${f}"
done
(But this code is from memory and untested, so inspect the convert
command about the actual resize-syntax, and try it in some sample directory on a few sample files first.)
Related videos on Youtube
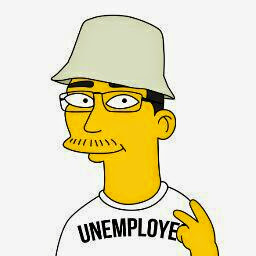
Tommy
Something for nothing. Bite me if you can score 9+ in a CPS Test.
Updated on September 18, 2022Comments
-
Tommy over 1 year
I want to resize all PNG files in
~/somefolder/
whose filenames are NOT started withtn_
to 50% of its original size and rename the output file with atn_
as its prefix and its original name. I know there's a convert command and I've already installed it. I guess it can be done via bash and some magic but I am quite new to Unix. I am using Mac OSX.What should I do?
-
Tommy about 9 yearsThanks. What is the
|| exit 1
for? -
Janis about 9 yearsA safety measure that let's the script exit (without doing anything) if the directory can't be entered. If that code would not be there it would start doing the subsequent operations in your current working directory, which may be undesired.
-
Ulrich Schwarz about 9 yearsNice use of
case
! (Though I personally would be worried about spaces in filenames and writecase "$f" in
just to be sure, regardless of whether it's needed there.) -
Janis about 9 years@Ulrich, FYI: The case construct has the "advantage" that it runs also in old Bourne shell, while the second version (the test operator with regexps) would not. WRT the variable quoting; they are indeed not necessary in the
case
argument (and also not even in the[[...]]
construct; but in the latter test construct I deliberately added them because some folks may use the old[...]
test command, and there they would be necessary).