Python 3 range Vs Python 2 range
Solution 1
Python 3 uses iterators for a lot of things where python 2 used lists.The docs give a detailed explanation including the change to range
.
The advantage is that Python 3 doesn't need to allocate the memory if you're using a large range iterator or mapping. For example
for i in range(1000000000): print(i)
requires a lot less memory in python 3. If you do happen to want Python to expand out the list all at once you can
list_of_range = list(range(10))
Solution 2
in python 2, range
is a built-in function. below is from the official python docs. it returns a list.
range(stop)
range(start, stop[, step])
This is a versatile function to create lists containing arithmetic progressions. It is most often used in for loops.
also you may check xrange
only existing in python 2. it returns xrange
object, mainly for fast iteration.
xrange(stop)
xrange(start, stop[, step])
This function is very similar to range(), but returns an xrange object instead of a list.
by the way, python 3 merges these two into one range
data type, working in a similar way of xrange
in python 2. check the docs.
Solution 3
Python 3 range()
function is equivalent to python 2 xrange()
function not range()
Explanation
In python 3 most function return Iterable objects not lists as in python 2 in order to save memory. Some of those are zip()
filter()
map()
including .keys .values .items()
dictionary methods
But iterable objects are not efficient if your trying to iterate several times so you can still use list()
method to convert them to lists
Solution 4
In python3, do
A = range(0,6)
A = list(A)
print(A)
You will get the same result.
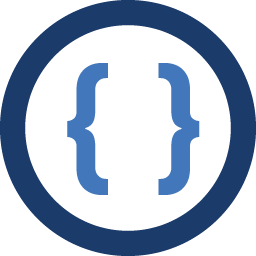
Admin
Updated on February 15, 2021Comments
-
Admin about 3 years
I recently started learning python 3.
In python 2 therange()
function can be used to assign list elements:>>> A = [] >>> A = range(0,6) >>> print A [0, 1, 2, 3, 4, 5]
But in python 3 the
range()
function outputs this:>>> A = [] >>> A = range(0,6) >>> print(A) range(0, 6)
Why is this happening?
Why did python do this change?
Is it a boon or a bane?