Use SystemVerilog parameters to decide which module to instantiate
If you want to conditionally instantiate a module, you need to use a generate
block.
generate
if (WORD == 1) begin
child_1 child();
end
if (WORD == 2) begin
child_2 child();
end
endgenerate
Below is a full working example. Note that it only accounts for the presence of child_1 and child_2. You cannot use the parameter as part of the module type name that you are instantiating. If you have N child modules and you don't want to explicitly enumerate them all in the generate block, you would probably need to create a helper macro.
BTW, this is valid Verilog code; it doesn't use any SystemVerilog features.
module child_1();
initial begin
$display("child_1 %m");
end
endmodule
module child_2();
initial begin
$display("child_2 %m");
end
endmodule
module parent();
parameter WORD = 1;
// Conditionally instantiate child_1 or child_2 depending
// depending on value of WORD parameter.
generate
if (WORD == 1) begin
child_1 child();
end
if (WORD == 2) begin
child_2 child();
end
endgenerate
endmodule
module top();
parent #(.WORD(1)) p1();
parent #(.WORD(2)) p2();
endmodule
Output from Incisive:
child_1 top.p1.genblk1.child
child_2 top.p2.genblk2.child
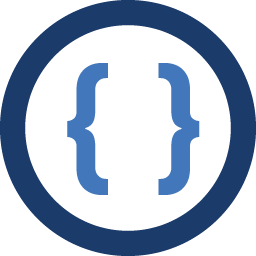
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
Is there a way to select the module I want to instantiate using the parameter values passed into the parent module? Example below
module parent (); parameter WORD = 1; child_`WORD child (); // obviously does not work endmodule
If
WORD == 1
, I would like to instantiate the child_1 module, forWORD == 2
, the child_2 module and so on. Surely, someone has had a need to do this before?